#include<iostream> using namespace std; class Cshape { public: void SetColor(int color) { m_nColor = color; } virtual void Display(void) { cout << "Cshape" << endl; } private: int m_nColor; }; class Crectangle : public Cshape { public: virtual void Display(void) { cout << "Crectangle" << endl; } }; class Ctriangle : public Cshape { virtual void Display(void) { cout << "Ctriangle" << endl; } }; class Cellipse :public Cshape { public: virtual void Display(void) { cout << "Cellipse" << endl; } }; int main() { Cshape obShape; Cellipse obEllipse; Ctriangle obTriangle; Crectangle obRectangle; Cshape * pShape[4]= { &obShape,&obEllipse, &obTriangle,& obRectangle }; for( int I= 0; I< 4; I++) pShape[I]->Display( ); }
时间: 2024-03-09 20:49:28 浏览: 26
这段代码与之前的代码非常相似,主要区别在于基类的 Display() 函数被声明为虚函数。这个小小的改动会产生很大的影响,因为它使得在运行时动态绑定函数成为可能。也就是说,当我们使用基类指针来调用一个虚函数时,实际上会调用指向对象的实际类型的函数。
在这个程序中,基类的 Display() 函数被声明为虚函数,因此在派生类中重载了 Display() 函数后,基类指针调用 Display() 函数时会根据指向对象的实际类型来动态绑定函数。因此,在运行时,分别调用了每个派生类的 Display() 函数,所以输出结果是:
```
Cshape
Cellipse
Ctriangle
Crectangle
```
这正是我们想要的输出结果。
相关问题
#include <iostream> #include<algorithm> #include<cmath> #include <queue> using namespace std;
#include <iostream>:这是C++标准库中的头文件,用于输入输出流操作,包括cin、cout等。
#include<algorithm>:这是C++标准库中的头文件,用于提供各种算法操作,如排序、查找等。
#include<cmath>:这是C++标准库中的头文件,用于提供数学函数操作,如绝对值、平方根等。
#include <queue>:这是C++标准库中的头文件,用于提供队列操作,包括入队、出队等。
using namespace std;:这是C++的命名空间声明,表示使用std命名空间,可以直接使用std中的函数和对象。
#include <iostream> #include <string> #include <stack> #include <vector> #include <iomanip> using namespace std; 逐行注释以上代码
这是一段C++的程序代码,使用了几个标准库的头文件。以下是逐行的注释:
```
#include <iostream> // 输入输出流库头文件
#include <string> // 字符串库头文件
#include <stack> // 栈库头文件
#include <vector> // 向量库头文件
#include <iomanip> // 格式控制库头文件
using namespace std; // 命名空间
```
这段程序代码导入了五个标准库的头文件,分别是iostream、string、stack、vector、iomanip,它们分别用于处理输入输出、字符串、栈、向量和格式控制。其中iostream是C++中最常用的输入输出流库,string是用于字符串操作的库,stack是用于栈数据结构的库,vector是用于动态数组的库,iomanip是用于格式化输出的库。
最后一行代码使用了命名空间std,这意味着所有的标准库函数和变量都在std命名空间中。如果不使用using namespace std,则需要在使用标准库函数时加上std::前缀。
相关推荐
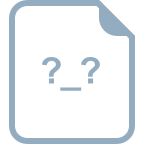
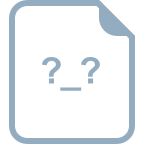














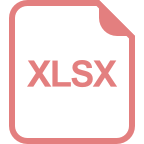