java调用http接口post请求提交json参数
时间: 2023-11-12 19:06:10 浏览: 100
可以使用Java提供的HttpURLConnection或者Apache HttpClient库来实现POST请求提交JSON参数。
使用HttpURLConnection实现POST请求提交JSON参数的示例代码如下:
```java
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.OutputStream;
public class HttpPostJson {
public static void main(String[] args) throws Exception {
String url = "http://example.com/api";
String json = "{\"username\":\"test\",\"password\":\"123456\"}";
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// 添加请求头
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
// 发送POST请求
con.setDoOutput(true);
OutputStream os = con.getOutputStream();
os.write(json.getBytes("UTF-8"));
os.flush();
os.close();
// 获取返回结果
int responseCode = con.getResponseCode();
System.out.println("Response Code : " + responseCode);
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// 打印返回结果
System.out.println(response.toString());
}
}
```
使用Apache HttpClient库实现POST请求提交JSON参数的示例代码如下:
```java
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.HttpResponse;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class HttpPostJson {
public static void main(String[] args) throws Exception {
String url = "http://example.com/api";
String json = "{\"username\":\"test\",\"password\":\"123456\"}";
HttpClient httpClient = HttpClientBuilder.create().build();
HttpPost request = new HttpPost(url);
// 设置请求头
request.setHeader("Content-Type", "application/json");
// 设置请求参数
StringEntity params = new StringEntity(json);
request.setEntity(params);
// 发送POST请求
HttpResponse response = httpClient.execute(request);
// 获取返回结果
BufferedReader rd = new BufferedReader(
new InputStreamReader(response.getEntity().getContent()));
StringBuffer result = new StringBuffer();
String line = "";
while ((line = rd.readLine()) != null) {
result.append(line);
}
// 打印返回结果
System.out.println(result.toString());
}
}
```
以上示例代码仅供参考,实际使用时需要根据自己的需求进行修改。
阅读全文
相关推荐
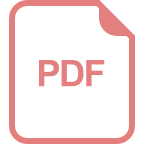
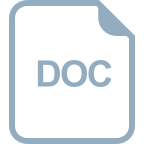

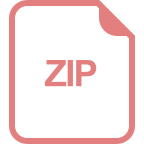
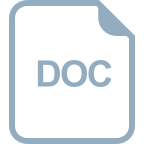







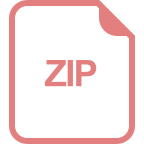
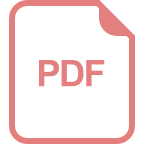


