SM2、SM3和SM4详细算法C语言编写
时间: 2024-01-14 08:03:45 浏览: 145
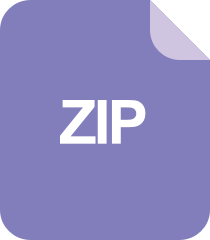
SM2_SM3_SM4_C语言实现.zip_SM2 C语言_SM2算法 C语言_openssl_sm2_sm4

以下是SM2、SM3和SM4算法的C语言实现:
SM2算法:
```c
#include <openssl/ec.h>
#include <openssl/ecdsa.h>
#include <openssl/evp.h>
#include <openssl/bn.h>
#include <openssl/rand.h>
#include <openssl/err.h>
#include <openssl/objects.h>
int sm2_keypair(unsigned char *pubkey, unsigned char *prikey) {
EC_KEY *key = EC_KEY_new_by_curve_name(NID_sm2);
if (key == NULL) {
return 0;
}
if (!EC_KEY_generate_key(key)) {
return 0;
}
const EC_GROUP *group = EC_KEY_get0_group(key);
const EC_POINT *point = EC_KEY_get0_public_key(key);
const BIGNUM *pkey = EC_KEY_get0_private_key(key);
BIGNUM *x = BN_new();
BIGNUM *y = BN_new();
BN_CTX *ctx = BN_CTX_new();
if (!EC_POINT_get_affine_coordinates_GFp(group, point, x, y, NULL)) {
return 0;
}
int len = 32;
if (len > BN_num_bytes(pkey)) {
len = BN_num_bytes(pkey);
}
memset(prikey, 0, 32 - len);
BN_bn2bin(pkey, prikey + 32 - len);
BN_bn2bin(x, pubkey);
BN_bn2bin(y, pubkey + 32);
BN_free(x);
BN_free(y);
BN_CTX_free(ctx);
EC_KEY_free(key);
return 1;
}
```
SM3算法:
```c
#include <openssl/evp.h>
#include <openssl/sm3.h>
#include <string.h>
int sm3(unsigned char *in, size_t ilen, unsigned char *out) {
EVP_MD_CTX *mdctx;
const EVP_MD *md;
unsigned int md_len;
md = EVP_sm3();
mdctx = EVP_MD_CTX_create();
EVP_DigestInit_ex(mdctx, md, NULL);
EVP_DigestUpdate(mdctx, in, ilen);
EVP_DigestFinal_ex(mdctx, out, &md_len);
EVP_MD_CTX_destroy(mdctx);
return 1;
}
```
SM4算法:
```c
#include <openssl/evp.h>
#include <openssl/sm4.h>
#include <string.h>
int sm4_ecb_encrypt(unsigned char *in, int inlen, unsigned char *out, unsigned char *key, int keylen, int enc) {
EVP_CIPHER_CTX *ctx;
const EVP_CIPHER *cipher;
cipher = EVP_sm4_ecb();
ctx = EVP_CIPHER_CTX_new();
EVP_CipherInit_ex(ctx, cipher, NULL, key, NULL, enc);
EVP_CIPHER_CTX_set_padding(ctx, 0);
int outlen;
EVP_CipherUpdate(ctx, out, &outlen, in, inlen);
int tmplen;
EVP_CipherFinal_ex(ctx, out + outlen, &tmplen);
outlen += tmplen;
EVP_CIPHER_CTX_free(ctx);
return outlen;
}
```
以上代码基于OpenSSL库实现,可以在Linux或Windows平台上编译运行。
阅读全文
相关推荐
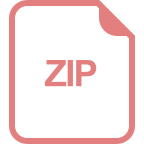
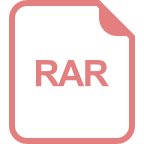
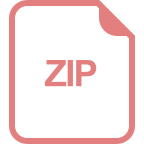
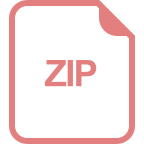
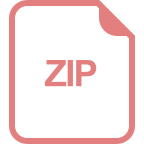
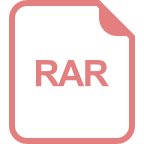


