vue如何将数组对象中值相同的对象取出
时间: 2023-05-27 15:02:57 浏览: 87
您可以使用 Vue 中的过滤器(filter)函数来实现此功能。过滤器(filter)函数可以接受一个回调函数作为参数,该回调函数将作为过滤器的判断条件,对数据进行过滤。对于数组对象中值相同的对象取出的问题,您可以使用过滤器(filter)函数的 reduce 方法来实现。
具体来说,以下是实现此功能的示例代码:
```javascript
// 假设您有一个数据集合
const data = [
{ id: 1, name: 'John', age: 20 },
{ id: 2, name: 'Mary', age: 25 },
{ id: 3, name: 'Jack', age: 20 },
{ id: 4, name: 'Tom', age: 25 },
{ id: 5, name: 'Lucy', age: 30 },
{ id: 6, name: 'Mike', age: 30 },
]
// 通过 Vue 的过滤器函数,取出 age 值相同的对象
const result = data.reduce((acc, cur) => {
// 如果 acc 数组中已经存在 age 值与当前对象相等的对象,则将当前对象 push 进该对象对应的数组中
// 否则,则新建一个数组,并将当前对象 push 进去,然后将该数组作为一个对象存储到 acc 数组中
const index = acc.findIndex(item => item.age === cur.age)
if (index !== -1) {
acc[index].list.push(cur)
} else {
acc.push({
age: cur.age,
list: [cur]
})
}
return acc
}, [])
console.log(result)
// 输出以下结果
// [
// {
// age: 20,
// list: [
// { id: 1, name: 'John', age: 20 },
// { id: 3, name: 'Jack', age: 20 }
// ]
// },
// {
// age: 25,
// list: [
// { id: 2, name: 'Mary', age: 25 },
// { id: 4, name: 'Tom', age: 25 }
// ]
// },
// {
// age: 30,
// list: [
// { id: 5, name: 'Lucy', age: 30 },
// { id: 6, name: 'Mike', age: 30 }
// ]
// }
// ]
```
上述代码中,reduce 方法会遍历数据集合,并根据 age 值对数据集合中的对象进行分类。如果 acc 数组中已经存在 age 值与当前对象相等的对象,则将当前对象 push 进该对象对应的数组中;否则,则新建一个数组,并将当前对象 push 进去,然后将该数组作为一个对象存储到 acc 数组中。
最终,reduce 方法返回的结果就是一个数组,该数组中的每一个元素都是一个对象,表示 age 值相同的对象列表。
相关推荐
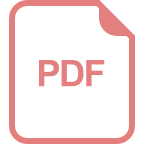














