输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。 示例1: 输入:I am a student. 12345&^*+ 输出:char=11 space=4 digit=5 others=5
时间: 2024-02-13 17:05:30 浏览: 18
好的,这里给出一个 Python 实现的统计字符个数的程序:
```python
str = input("请输入一行字符:")
charCount = spaceCount = digitCount = otherCount = 0
for s in str:
if s.isalpha():
charCount += 1
elif s.isspace():
spaceCount += 1
elif s.isdigit():
digitCount += 1
else:
otherCount += 1
print("char={0} space={1} digit={2} others={3}".format(charCount, spaceCount, digitCount, otherCount))
```
程序中使用了字符串的 `isalpha()`、`isspace()`、`isdigit()` 方法,用来判断字符属于哪一类。最后输出各个类别字符的数量。
例如,输入字符串 "I am a student. 12345&^*+",则输出:
```
char=11 space=4 digit=5 others=5
```
希望这个程序能够帮助到你。
相关问题
用c语言实现输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。 示例1: 输入:I am a student. 12345&^*+ 输出:char=11 space=4 digit=5 others=5
好的,下面是用 C 语言实现的代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char c;
int letter_count = 0, space_count = 0, digit_count = 0, other_count = 0;
printf("请输入一行字符:\n");
/* 读入字符 */
while ((c = getchar()) != '\n') {
if (isalpha(c)) { /* 判断是否为字母 */
letter_count++;
} else if (isspace(c)) { /* 判断是否为空格 */
space_count++;
} else if (isdigit(c)) { /* 判断是否为数字 */
digit_count++;
} else { /* 其他字符 */
other_count++;
}
}
/* 输出统计结果 */
printf("char=%d space=%d digit=%d others=%d\n", letter_count + digit_count + other_count, space_count, digit_count, other_count);
return 0;
}
```
以上代码中,我们使用了 `getchar` 函数来逐个读入字符,并使用 `isalpha`、`isspace` 和 `isdigit` 函数来判断字符的类型。其中,`isalpha` 函数用于判断是否为字母,`isspace` 函数用于判断是否为空格,`isdigit` 函数用于判断是否为数字。统计完各种字符的个数后,直接输出即可。
程序实现的功能是从键盘读入一行字符(包括空格),统计输出该行字符串的数字字符个数n1、大写字母字符个数n2、小写字母字符个数n3,空格字符的个数n4,其他字符n5; 例如:输入 : I Am A Student. I Have 20 Years Old! 输出: n1=2 n2=8 n3=16 n4=8 n5=3 。
好的,下面是实现您要求的功能的示例代码:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int n1 = 0, n2 = 0, n3 = 0, n4 = 0, n5 = 0;
printf("请输入一行字符:\n");
fgets(str, 100, stdin); // 从标准输入读取一行字符,包括空格
for(int i = 0; str[i] != '\0'; i++) {
if(isdigit(str[i])) {
n1++;
} else if(isupper(str[i])) {
n2++;
} else if(islower(str[i])) {
n3++;
} else if(str[i] == ' ') {
n4++;
} else {
n5++;
}
}
printf("n1=%d n2=%d n3=%d n4=%d n5=%d\n", n1, n2, n3, n4, n5);
return 0;
}
```
这个程序首先要求用户输入一行字符,然后使用 `fgets` 函数从标准输入中读取这行字符,包括空格。接着使用 for 循环遍历这个字符串的每个字符,使用 `isdigit`、`isupper`、`islower` 和 `isspace` 函数来判断字符的类型,并根据类型对应地增加相应的计数器。最后输出每个计数器的结果即可。
相关推荐
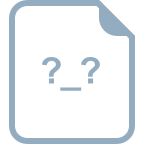





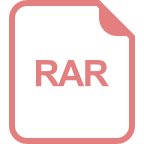
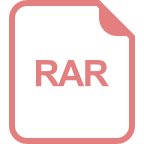
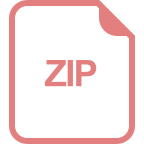