手写LinkedList的add、get、size、indexOf、remove方法
时间: 2023-07-10 09:33:11 浏览: 112
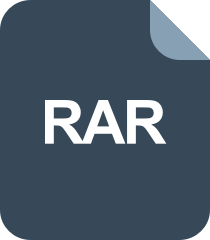
添加,删除,插入链表
下面是一个手写LinkedList的示例代码,包含了add、get、size、indexOf、remove方法:
```java
public class MyLinkedList<E> {
private Node<E> head;
private int size;
private static class Node<E> {
E data;
Node<E> next;
public Node(E data) {
this.data = data;
this.next = null;
}
}
public void add(E element) {
Node<E> newNode = new Node<>(element);
if (head == null) {
head = newNode;
} else {
Node<E> current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
size++;
}
public E get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index: " + index + ", Size: " + size);
}
Node<E> current = head;
for (int i = 0; i < index; i++) {
current = current.next;
}
return current.data;
}
public int size() {
return size;
}
public int indexOf(E element) {
int index = 0;
Node<E> current = head;
while (current != null) {
if (current.data.equals(element)) {
return index;
}
index++;
current = current.next;
}
return -1;
}
public boolean remove(E element) {
if (head == null) {
return false;
}
if (head.data.equals(element)) {
head = head.next;
size--;
return true;
}
Node<E> current = head;
while (current.next != null) {
if (current.next.data.equals(element)) {
current.next = current.next.next;
size--;
return true;
}
current = current.next;
}
return false;
}
}
```
解释:
- 首先,我们定义了一个Node类表示链表的节点。每个节点包含一个存储元素的data变量和一个指向下一个节点的next指针。
- 我们还定义了一个头节点head和一个size变量表示链表的大小。
- add方法用于在链表末尾添加一个元素。首先,创建一个新的节点,然后将其添加到链表的末尾。如果链表为空,将新节点设置为头节点。最后,将链表的大小增加1。
- get方法用于获取指定下标的元素。首先,检查下标是否越界,如果是,则抛出IndexOutOfBoundsException异常。然后,从头节点开始遍历链表,直到找到指定下标的节点。最后,返回该节点的元素。
- size方法用于获取链表的大小。它只需返回之前维护的size变量即可。
- indexOf方法用于查找指定元素在链表中的下标。从头节点开始遍历链表,直到找到包含指定元素的节点。如果找到了该元素,则返回其下标值。如果遍历完整个链表后仍然没有找到元素,那么就返回-1,表示该元素不存在于链表中。
- remove方法用于从链表中删除指定元素。首先,检查链表是否为空,如果是,则直接返回false。然后,检查头节点是否包含指定元素,如果是,则将头节点设置为下一个节点。否则,从第二个节点开始遍历链表,直到找到包含指定元素的节点。如果找到了该元素,则将其从链表中删除,并将链表的大小减1。如果遍历完整个链表后仍然没有找到元素,那么就返回false。
阅读全文
相关推荐
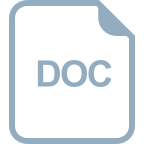



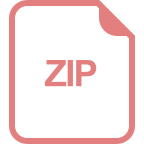
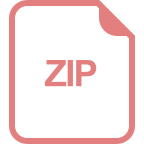
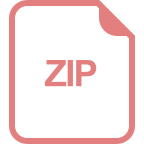
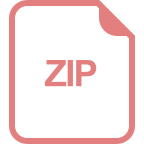



