c++如何调用python程序
时间: 2023-09-20 07:12:32 浏览: 6
C++可以通过调用Python的解释器来运行Python程序,具体步骤如下:
1. 安装Python解释器并配置环境变量。
2. 在C++中使用system或popen函数调用Python程序。其中system函数可以直接执行Python程序,popen函数可以在C++程序中调用Python程序并获取Python程序返回结果。
例如,下面是一个使用system函数调用Python程序的示例代码:
```c++
#include <stdlib.h>
int main()
{
// 调用Python程序
system("python3 /path/to/python/file.py");
return 0;
}
```
注意,这种方式可能存在安全隐患,因为system函数会执行任何命令,包括可能会导致系统崩溃或数据损坏的恶意命令。因此,建议使用popen函数来调用Python程序并获取结果。
相关问题
c++调用python程序
要在 C++ 中调用 Python 程序,可以使用 Python 的 C API。具体来说,可以按照以下步骤进行操作:
1. 在 C++ 代码中包含 Python.h 头文件。
2. 使用 Py_Initialize() 函数初始化 Python 解释器。
3. 使用 PyRun_SimpleString() 函数或 PyRun_File() 函数执行 Python 代码。
4. 使用 Py_Finalize() 函数关闭 Python 解释器。
以下是一个简单的例子,演示如何在 C++ 中调用 Python 程序:
```c++
#include <Python.h>
int main()
{
// 初始化 Python 解释器
Py_Initialize();
// 执行 Python 代码
PyRun_SimpleString("print('Hello, World!')");
// 关闭 Python 解释器
Py_Finalize();
return 0;
}
```
在上面的例子中,我们使用 Py_Initialize() 函数初始化 Python 解释器,使用 PyRun_SimpleString() 函数执行 Python 代码,然后使用 Py_Finalize() 函数关闭 Python 解释器。
当然,如果需要传递参数给 Python 程序,可以使用 PyRun_SimpleString() 函数或 PyRun_File() 函数的参数来指定 Python 程序文件名和参数。
c++ 调用python文件的程序
你可以使用Python提供的subprocess模块来调用Python文件,具体代码如下:
```c++
#include <iostream>
#include <string>
#include <cstdio>
using namespace std;
int main() {
string cmd = "python /path/to/your/python/script.py";
FILE* pipe = popen(cmd.c_str(), "r");
if (!pipe) {
cout << "执行Python脚本失败!" << endl;
return 1;
}
char buffer[128];
while (!feof(pipe)) {
if (fgets(buffer, 128, pipe)) {
cout << buffer;
}
}
pclose(pipe);
return 0;
}
```
其中,`/path/to/your/python/script.py`指的是你要调用的Python文件的路径。这段代码会在C++程序中执行一个命令行,然后调用Python文件并输出Python脚本的输出。
相关推荐
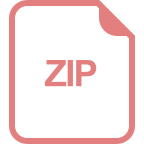
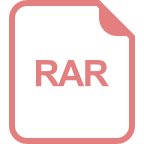
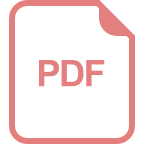








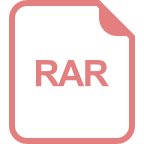
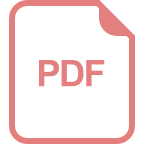
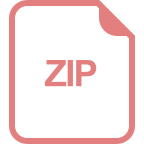
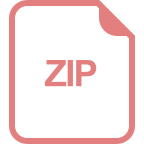
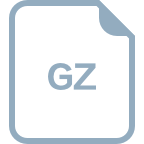