批量读取文件夹里的txt文件,根据停用词词表以及词表使用最大逆向算法进行分词,词表说明了哪些字的组合可以被算作一个词,停用词表说明哪些没有实意的词可以去掉,其中词表和停用词表均为excel文件,将分词结果以同名文件储存在另一个文件夹里,同时将分词结果进行词频统计并保存在另一个文件夹里
时间: 2024-03-20 12:43:49 浏览: 113
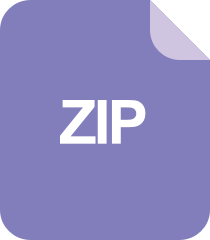
文本分词常用中文停用词表
好的,这是一个比较复杂的任务,需要用到一些Python中的库和技术。下面是实现的大致步骤:
1. 读取excel文件中的词表和停用词表到内存中,存储为列表。
2. 遍历文件夹中的txt文件,逐个读取文件并进行分词。
3. 对于每个txt文件,先将其内容进行清洗,例如去除标点符号、数字等,得到一个纯文本字符串。
4. 使用最大逆向算法进行分词,得到一个分词后的词列表。
5. 遍历分词后的词列表,去除停用词,并根据词表将词组合成新的词。
6. 将分词结果写入同名文件夹中的文件中,并将分词结果进行词频统计并保存在另一个文件夹里。
下面是代码实现的一个框架,你可以根据需要进行修改和完善:
```python
import os
import xlrd
from collections import Counter
# 读取词表和停用词表
def read_excel(file_path):
words = []
workbook = xlrd.open_workbook(file_path)
sheet = workbook.sheet_by_index(0)
for i in range(sheet.nrows):
words.append(sheet.cell_value(i, 0))
return words
# 进行分词
def segment(text, stopwords, wordlist):
# TODO: 最大逆向算法分词
# 清洗文本
def clean_text(text):
# TODO: 去除标点符号、数字等
# 对单个文件进行分词和词频统计
def process_file(file_path, stopwords, wordlist, output_dir):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
text = clean_text(text)
words = segment(text, stopwords, wordlist)
words_count = Counter(words)
file_name = os.path.basename(file_path)
output_path = os.path.join(output_dir, file_name)
with open(output_path, 'w', encoding='utf-8') as f:
for word, count in words_count.items():
f.write(f"{word}\t{count}\n")
# 对文件夹中的所有文件进行分词和词频统计
def process_dir(input_dir, output_dir, stopwords_path, wordlist_path):
stopwords = read_excel(stopwords_path)
wordlist = read_excel(wordlist_path)
for file_name in os.listdir(input_dir):
if file_name.endswith('.txt'):
file_path = os.path.join(input_dir, file_name)
process_file(file_path, stopwords, wordlist, output_dir)
# 调用函数进行处理
input_dir = 'input_folder'
output_dir = 'output_folder'
stopwords_path = 'stopwords.xlsx'
wordlist_path = 'wordlist.xlsx'
process_dir(input_dir, output_dir, stopwords_path, wordlist_path)
```
需要注意的是,上述代码中的分词算法只是一个占位符,你需要自己实现最大逆向算法分词的代码。另外,如果你的文本比较大,建议使用分段分词的方式,避免内存占用过大。
阅读全文
相关推荐
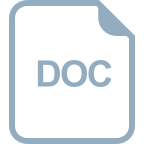
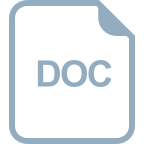



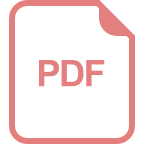
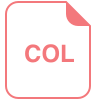


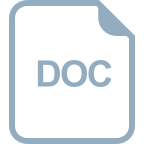
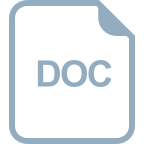