linux C++压缩解压文件
时间: 2023-12-02 13:03:11 浏览: 225
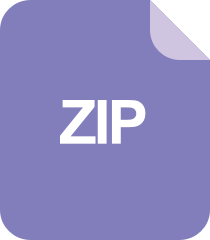
linux环境下利用zlib库实现压缩目录的c++程序

要在 Linux C++ 中进行文件压缩和解压缩,可以使用 zlib 库。该库提供了一组函数,可以在 C++ 中使用。
以下是一个简单的示例代码,展示了如何使用 zlib 库进行文件压缩和解压缩:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <zlib.h>
// 压缩文件
void compressFile(const std::string& srcFile, const std::string& dstFile)
{
std::ifstream ifs(srcFile.c_str(), std::ios::binary);
std::ofstream ofs(dstFile.c_str(), std::ios::binary);
if (!ifs || !ofs) {
std::cerr << "open file failed" << std::endl;
return;
}
// 初始化 zlib
z_stream strm;
strm.zalloc = Z_NULL;
strm.zfree = Z_NULL;
strm.opaque = Z_NULL;
if (deflateInit(&strm, Z_DEFAULT_COMPRESSION) != Z_OK) {
std::cerr << "deflateInit failed" << std::endl;
return;
}
// 压缩数据
char in[1024];
char out[1024];
do {
ifs.read(in, sizeof(in));
strm.avail_in = ifs.gcount();
strm.next_in = reinterpret_cast<Bytef*>(in);
do {
strm.avail_out = sizeof(out);
strm.next_out = reinterpret_cast<Bytef*>(out);
deflate(&strm, Z_FINISH);
ofs.write(out, sizeof(out) - strm.avail_out);
} while (strm.avail_out == 0);
} while (!ifs.eof());
// 结束压缩
deflateEnd(&strm);
}
// 解压文件
void uncompressFile(const std::string& srcFile, const std::string& dstFile)
{
std::ifstream ifs(srcFile.c_str(), std::ios::binary);
std::ofstream ofs(dstFile.c_str(), std::ios::binary);
if (!ifs || !ofs) {
std::cerr << "open file failed" << std::endl;
return;
}
// 初始化 zlib
z_stream strm;
strm.zalloc = Z_NULL;
strm.zfree = Z_NULL;
strm.opaque = Z_NULL;
if (inflateInit(&strm) != Z_OK) {
std::cerr << "inflateInit failed" << std::endl;
return;
}
// 解压数据
char in[1024];
char out[1024];
do {
ifs.read(in, sizeof(in));
strm.avail_in = ifs.gcount();
strm.next_in = reinterpret_cast<Bytef*>(in);
do {
strm.avail_out = sizeof(out);
strm.next_out = reinterpret_cast<Bytef*>(out);
inflate(&strm, Z_NO_FLUSH);
ofs.write(out, sizeof(out) - strm.avail_out);
} while (strm.avail_out == 0);
} while (!ifs.eof());
// 结束解压
inflateEnd(&strm);
}
int main()
{
const std::string srcFile = "test.txt";
const std::string dstFile = "test.gz";
// 压缩文件
compressFile(srcFile, dstFile);
// 解压文件
uncompressFile(dstFile, "output.txt");
return 0;
}
```
在上面的代码中,compressFile 函数用于压缩文件,uncompressFile 函数用于解压文件。这两个函数都包含了对 zlib 库的初始化、数据处理和结束操作。请注意,这里使用的是 gzip 压缩格式,因此压缩文件的扩展名为 .gz。
阅读全文
相关推荐

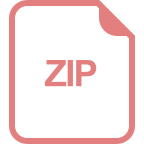
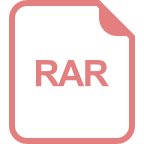

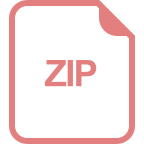
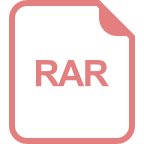
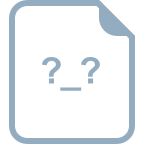
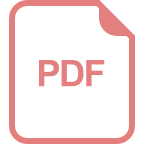
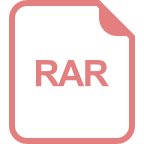
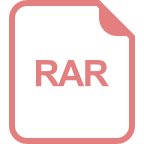
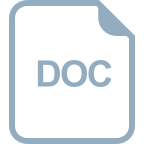
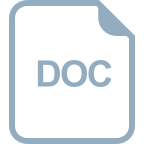
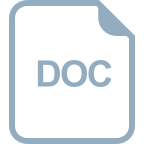
