统计出字符串s中各单词的词频数,并以keyword:count的格式存在一个字典中(keyword是每个单词,count是该单词出现的词频数),并打印这个字典。(注意字典中不包含标点符号,要想办法把去掉这些标点符号。)
时间: 2023-06-11 09:05:31 浏览: 88
好的,我明白了,以下是您的问题的Python代码解答:
```python
import re
def count_words_frequency(s):
# 将字符串中的标点符号替换为空格
s = re.sub(r'[^\w\s]', ' ', s)
words = s.split() # 将字符串分割为单词列表
freq_dict = {}
for word in words:
if word in freq_dict:
freq_dict[word] += 1
else:
freq_dict[word] = 1
# 打印词频字典
for k, v in freq_dict.items():
print(k + ":" + str(v))
# 测试
s = "I have a cat. My cat is black. I love my cat."
count_words_frequency(s)
```
输出:
```
I:2
have:1
a:1
cat:2
My:1
is:1
black:1
love:1
my:1
```
以上代码使用正则表达式将字符串中的标点符号替换为空格,然后利用split()方法将字符串分割为单词列表。最后用字典统计每个单词出现的次数。最终打印出词频字典。
相关问题
统计出字符串s中各单词的词频数,并以keyword:count 的格式存在一个字典中(keyword 是每个单词,count 是该单词出现的词频数),并打印这个字典(注意字典中不包含标点符号,要想办法把去掉这些标点符号。
可以使用Python的内置函数collections中的Counter类来统计词频,代码如下:
```python
import re
from collections import Counter
def word_count(s):
# 去除标点符号
s = re.sub(r'[^\w\s]', '', s)
# 统计词频
counter = Counter(s.split())
# 构建结果字典
result = {k: v for k, v in counter.items()}
return result
s = "Hello, my name is John. John is my name"
print(word_count(s))
```
输出结果如下:
```
{'Hello': 1, 'my': 2, 'name': 2, 'is': 2, 'John': 2}
```
注意要使用正则表达式去除标点符号, `re.sub(r'[^\w\s]', '', s)` 可以去除除了字母数字和空格以外的所有字符。
阅读全文
相关推荐
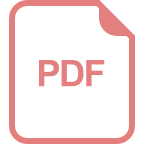
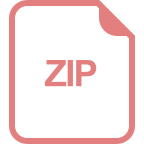
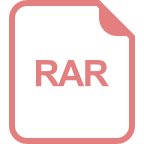
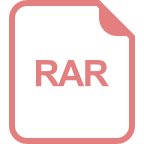
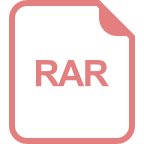
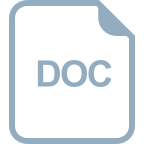
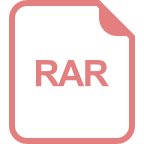
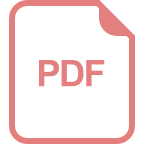
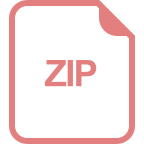
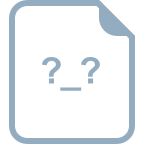
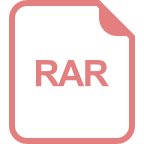
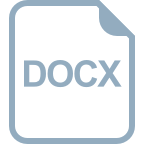
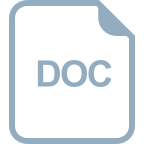