用python写一个机票预订系统
时间: 2023-11-27 19:06:23 浏览: 60
好的,机票预订系统可以分为以下几个部分:
1. 用户登录/注册模块
2. 航班查询模块
3. 航班订购模块
4. 订单管理模块
下面是一个简单的代码示例:
1. 用户登录/注册模块
```
import json
# 用户注册
def register(username, password):
with open('users.json', 'r') as f:
users = json.load(f)
if username in users:
return False
users[username] = password
with open('users.json', 'w') as f:
json.dump(users, f)
return True
# 用户登录
def login(username, password):
with open('users.json', 'r') as f:
users = json.load(f)
if username not in users or users[username] != password:
return False
return True
```
2. 航班查询模块
```
import csv
# 查询航班信息
def search_flight(departure, destination, date):
with open('flights.csv', 'r') as f:
flights = csv.reader(f)
for flight in flights:
if flight[0] == departure and flight[1] == destination and flight[2] == date:
return flight
return None
```
3. 航班订购模块
```
import random
# 生成订单号
def generate_order_id():
return str(random.randint(10000000, 99999999))
# 订购航班
def book_flight(username, flight):
order_id = generate_order_id()
with open('orders.json', 'r') as f:
orders = json.load(f)
if username not in orders:
orders[username] = []
orders[username].append({
'order_id': order_id,
'flight': flight
})
with open('orders.json', 'w') as f:
json.dump(orders, f)
return order_id
```
4. 订单管理模块
```
# 获取用户订单
def get_orders(username):
with open('orders.json', 'r') as f:
orders = json.load(f)
if username not in orders:
return []
return orders[username]
# 取消订单
def cancel_order(username, order_id):
with open('orders.json', 'r') as f:
orders = json.load(f)
if username not in orders:
return False
for order in orders[username]:
if order['order_id'] == order_id:
orders[username].remove(order)
with open('orders.json', 'w') as f:
json.dump(orders, f)
return True
return False
```
以上是一个简单的机票预订系统代码示例,仅供参考。实际开发中需要根据具体需求进行修改和完善。
相关推荐
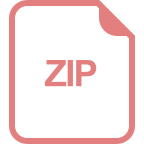
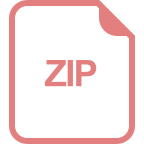
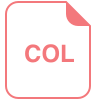














