使用java语言设计一个基于TCP的文件上传功能,将文件从客户端上传到指定服务器的可行程序。 并实现文件传输完成后服务器给客户端发送“文件上传成功”的信息。
时间: 2024-03-08 15:51:04 浏览: 59
以下是一个基于TCP协议的Java程序,实现了将文件从客户端上传到指定服务器的功能,并在文件传输完成后向客户端发送“文件上传成功”的信息。
服务端代码:
```java
import java.io.*;
import java.net.*;
public class FileServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = null;
try {
serverSocket = new ServerSocket(9999);
} catch (IOException e) {
System.err.println("Could not listen on port: 9999.");
System.exit(1);
}
Socket clientSocket = null;
try {
System.out.println("Waiting for client...");
clientSocket = serverSocket.accept();
System.out.println("Client connected: " + clientSocket.getInetAddress().getHostName());
} catch (IOException e) {
System.err.println("Accept failed.");
System.exit(1);
}
InputStream in = clientSocket.getInputStream();
OutputStream out = null;
try {
out = new FileOutputStream("server-file.txt");
} catch (FileNotFoundException e) {
System.err.println("File not found!");
System.exit(1);
}
byte[] bytes = new byte[1024];
int count;
while ((count = in.read(bytes)) > 0) {
out.write(bytes, 0, count);
}
out.close();
in.close();
clientSocket.close();
serverSocket.close();
PrintWriter pw = new PrintWriter(clientSocket.getOutputStream(), true);
pw.println("File uploaded successfully!");
System.out.println("File uploaded successfully!");
}
}
```
客户端代码:
```java
import java.io.*;
import java.net.*;
public class FileClient {
public static void main(String[] args) throws IOException {
Socket socket = null;
try {
socket = new Socket("localhost", 9999);
System.out.println("Connected to server: " + socket.getInetAddress().getHostName());
} catch (UnknownHostException e) {
System.err.println("Don't know about host: localhost.");
System.exit(1);
} catch (IOException e) {
System.err.println("Couldn't get I/O for the connection to: localhost.");
System.exit(1);
}
File file = new File("client-file.txt");
FileInputStream in = null;
try {
in = new FileInputStream(file);
} catch (FileNotFoundException e) {
System.err.println("File not found!");
System.exit(1);
}
OutputStream out = socket.getOutputStream();
byte[] bytes = new byte[1024];
int count;
while ((count = in.read(bytes)) > 0) {
out.write(bytes, 0, count);
}
out.close();
in.close();
socket.close();
BufferedReader br = new BufferedReader(new InputStreamReader(socket.getInputStream()));
String message = br.readLine();
System.out.println("Message from server: " + message);
}
}
```
在客户端和服务端的代码中,文件名和端口号可以根据具体需求进行修改。
阅读全文
相关推荐
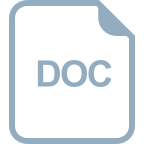
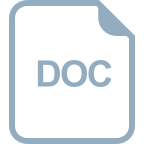
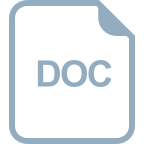
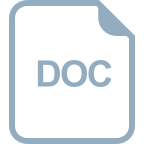
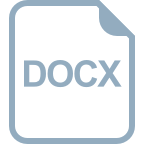
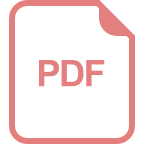
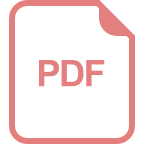
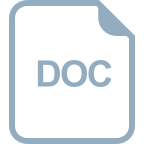
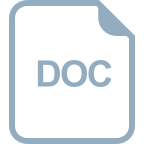
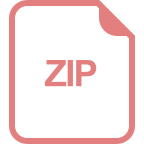
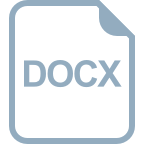
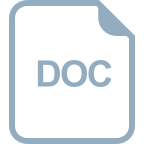
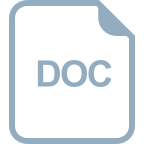
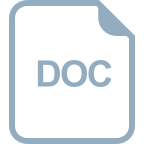
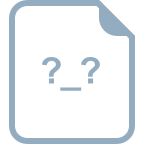