查询指定纯文本文件(可外部调用者设定)中指定字符串(可外部调用者设定)的位置(行号、列号),匹配行原始内容,字符串出现次数,并将用户输入参数和输出结果存放到mysql数据库
时间: 2024-05-13 14:13:33 浏览: 68
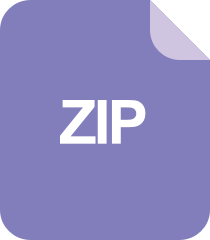
从数据库读取并解析大文本.zip_SQL 查询大文本_sql查询时加上行号
中。
伪代码:
1. 从外部调用者获取纯文本文件路径和指定字符串。
2. 打开纯文本文件,遍历每一行。
3. 对于每一行,使用正则表达式查找指定字符串出现的位置和次数。
4. 如果找到了指定字符串,记录行号、列号和出现次数,并将匹配行的原始内容存入列表中。
5. 将所有匹配结果存入字典中。
6. 将用户输入参数、输出结果和当前时间存入mysql数据库中。
实际代码:
```python
import re
import pymysql
from datetime import datetime
# 获取用户输入参数
file_path = input("请输入纯文本文件路径:")
search_str = input("请输入要查找的字符串:")
# 连接mysql数据库
db = pymysql.connect("localhost", "root", "password", "test")
cursor = db.cursor()
# 创建表格
sql = """CREATE TABLE IF NOT EXISTS `search_result` (
`id` INT UNSIGNED AUTO_INCREMENT PRIMARY KEY,
`file_path` VARCHAR(100) NOT NULL,
`search_str` VARCHAR(100) NOT NULL,
`line_num` INT NOT NULL,
`col_num` INT NOT NULL,
`match_content` VARCHAR(100) NOT NULL,
`match_count` INT NOT NULL,
`search_time` DATETIME NOT NULL)"""
cursor.execute(sql)
# 打开纯文本文件,遍历每一行
with open(file_path, "r") as f:
lines = f.readlines()
match_results = {}
for i, line in enumerate(lines):
# 使用正则表达式查找指定字符串出现的位置和次数
match = re.findall(search_str, line)
if match:
col_num = line.find(match[0]) + 1
match_count = len(match)
# 记录行号、列号和出现次数,并将匹配行的原始内容存入列表中
match_results[i+1] = {"col_num": col_num, "match_count": match_count, "match_content": line.strip()}
# 将所有匹配结果存入字典中
if match_results:
for line_num, match_result in match_results.items():
col_num = match_result["col_num"]
match_content = match_result["match_content"]
match_count = match_result["match_count"]
# 将用户输入参数、输出结果和当前时间存入mysql数据库中
search_time = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
sql = "INSERT INTO `search_result` (`file_path`, `search_str`, `line_num`, `col_num`, `match_content`, `match_count`, `search_time`) VALUES ('%s', '%s', '%d', '%d', '%s', '%d', '%s')" % (file_path, search_str, line_num, col_num, match_content, match_count, search_time)
cursor.execute(sql)
db.commit()
# 输出匹配结果
print("在第%d行第%d列找到了%s,出现了%d次。" % (line_num, col_num, search_str, match_count))
print("匹配行的原始内容为:", match_content)
else:
print("未找到%s。" % search_str)
# 关闭数据库连接
db.close()
```
阅读全文
相关推荐
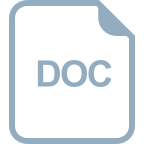
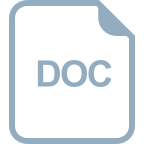
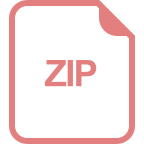
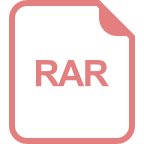
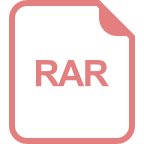
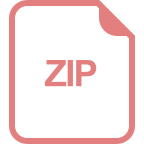
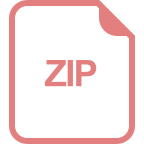
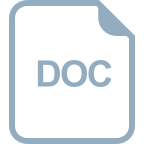
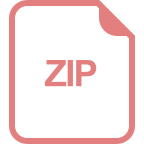
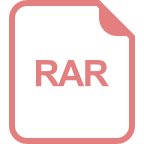
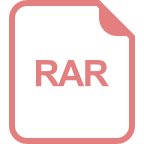
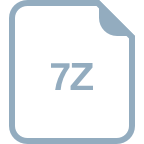
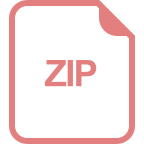
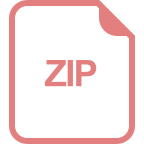
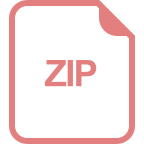