pthread_kill的信号处理函数
时间: 2023-09-13 15:10:31 浏览: 245
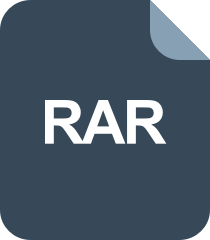
test_pthread_kill测试程序_
当我们调用pthread_kill函数向一个线程发送信号时,如果该线程没有设置对该信号的处理函数,则该信号会被默认处理。如果该线程设置了对该信号的处理函数,则该处理函数会被调用。
对于线程的信号处理函数,有以下几个注意点:
1. 信号处理函数需要使用sigaction函数进行注册,而不能使用signal函数;
2. 信号处理函数需要是一个void类型的函数,参数为int类型;
3. 信号处理函数需要在一定时间内执行完毕,否则可能会被操作系统强制终止;
4. 在信号处理函数中,为了避免竞态条件,需要避免使用非线程安全的函数和数据结构。
例如,以下是一个简单的线程信号处理函数的示例:
```c
void sig_handler(int signum) {
printf("Received signal %d\n", signum);
// do something
}
int main() {
pthread_t tid;
int ret;
// 创建线程
ret = pthread_create(&tid, NULL, thread_func, NULL);
if (ret != 0) {
printf("pthread_create error\n");
return -1;
}
// 向线程发送信号
ret = pthread_kill(tid, SIGINT);
if (ret != 0) {
printf("pthread_kill error\n");
return -1;
}
// 等待线程退出
ret = pthread_join(tid, NULL);
if (ret != 0) {
printf("pthread_join error\n");
return -1;
}
return 0;
}
void *thread_func(void *arg) {
// 注册信号处理函数
struct sigaction act;
act.sa_handler = sig_handler;
sigemptyset(&act.sa_mask);
act.sa_flags = 0;
sigaction(SIGINT, &act, NULL);
// 等待信号
while (1) {
sleep(1);
}
return NULL;
}
```
在该示例中,我们创建了一个线程,并向该线程发送了一个SIGINT信号。该线程的信号处理函数简单地打印了接收到的信号编号。注意,该线程的信号处理函数需要先使用sigaction函数进行注册。
阅读全文
相关推荐
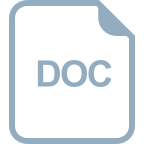
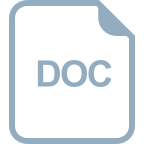

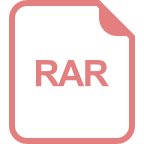













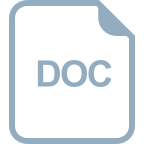