给出逻辑回归模型算法,包含逻辑回归模型的参数估计算法,并可视化结果,画出决策边界,用python实现
时间: 2023-07-11 07:50:35 浏览: 93
好的,我会给出逻辑回归模型算法及其参数估计算法,并用Python实现可视化结果。
逻辑回归模型是一种分类模型,它的目标是根据输入数据的特征来预测输出数据的类别。它的基本思想是将输入数据与一个权重向量进行加权求和,然后将结果传递给一个激活函数(如sigmoid函数),该函数将输出值转换为概率值。
逻辑回归模型的参数估计算法是最大似然估计。该算法的目标是找到最优的权重向量,使得模型产生的输出概率最大。具体来说,对于给定的输入样本,我们可以计算其真实类别的概率,然后最大化这些概率的乘积。最大化这个乘积的过程可以通过梯度下降算法来完成。
下面是Python实现逻辑回归模型的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
class LogisticRegression:
def __init__(self, learning_rate=0.01, num_iterations=1000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def fit(self, X, y):
m, n = X.shape
self.theta = np.zeros((n, 1))
self.costs = []
for i in range(self.num_iterations):
z = np.dot(X, self.theta)
h = self.sigmoid(z)
cost = (-1 / m) * np.sum(y * np.log(h) + (1 - y) * np.log(1 - h))
grad = (1 / m) * np.dot(X.T, (h - y))
self.theta -= self.learning_rate * grad
self.costs.append(cost)
def predict(self, X):
z = np.dot(X, self.theta)
h = self.sigmoid(z)
return (h > 0.5).astype(int)
def plot_decision_boundary(self, X, y):
x_min, x_max = X[:, 0].min() - 0.5, X[:, 0].max() + 0.5
y_min, y_max = X[:, 1].min() - 0.5, X[:, 1].max() + 0.5
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.01), np.arange(y_min, y_max, 0.01))
Z = self.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.4)
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=plt.cm.binary)
# 测试数据
X = np.array([[1, 2], [2, 3], [3, 4], [4, 5], [5, 6], [6, 7], [7, 8], [8, 9], [9, 10], [10, 11]])
y = np.array([0, 0, 0, 0, 1, 1, 1, 1, 1, 1])
# 训练模型
model = LogisticRegression()
model.fit(X, y)
# 可视化结果
model.plot_decision_boundary(X, y)
plt.show()
```
该代码实现了一个简单的逻辑回归模型,使用随机生成的数据进行了测试,并可视化了决策边界。您可以根据您的需求进行修改和优化。
相关推荐
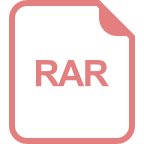
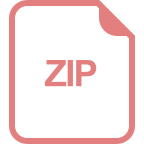
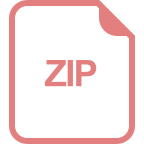
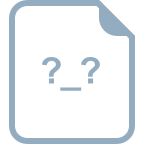
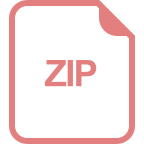
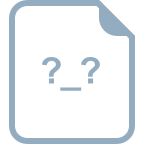
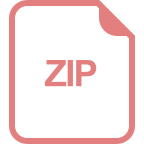
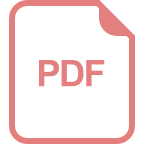
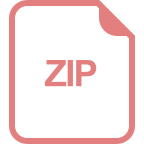
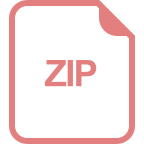
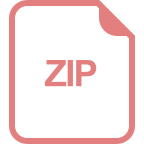
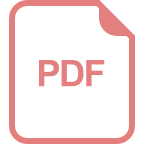
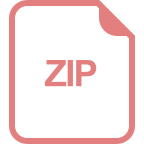
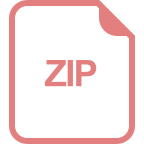