zabbix利用java通过其api接口创建拓扑图的节点和连线
时间: 2023-07-29 19:08:03 浏览: 46
以下是使用 Java 调用 Zabbix API 接口创建拓扑图节点和连线的大致步骤:
1. 首先,需要设置好 Java 开发环境,并引入 Zabbix API 的 Java Client,例如 zabbix-java-client。
2. 使用 Zabbix API 获取需要创建拓扑图节点和连线的主机和设备信息,例如主机 ID、名称、IP 地址等。
3. 使用 Zabbix API 的 graph.create 方法创建拓扑图,传入参数包括拓扑图名称、宽高等。
4. 使用 Zabbix API 的 graph.update 方法更新拓扑图,传入参数包括节点和连线的信息,例如节点 ID、名称、类型、坐标等。
5. 调用 Zabbix API 的 graph.get 方法获取拓扑图信息,可以查看是否创建成功。
下面是一个简单的 Java 代码示例,用于创建一个名为 "My Topology" 的拓扑图,并在其中添加一个名为 "My Host" 的主机节点和一个名为 "My Device" 的设备节点,并用一条连线将它们连接起来:
```java
import com.github.zabbixjavaclient.ZabbixApi;
import com.github.zabbixjavaclient.bean.GraphItem;
import com.github.zabbixjavaclient.bean.GraphType;
import com.github.zabbixjavaclient.bean.Request;
import com.github.zabbixjavaclient.bean.RequestBuilder;
public class CreateTopology {
public static void main(String[] args) throws Exception {
// 设置 Zabbix API 连接信息
String url = "http://localhost/zabbix/api_jsonrpc.php";
String username = "admin";
String password = "zabbix";
ZabbixApi zabbixApi = new ZabbixApi(url);
zabbixApi.login(username, password);
// 创建拓扑图
Request createGraphRequest = RequestBuilder.newBuilder()
.method("graph.create")
.paramEntry("name", "My Topology")
.paramEntry("width", 800)
.paramEntry("height", 600)
.build();
String graphId = zabbixApi.call(createGraphRequest).getResult().getGraphids().get(0);
// 创建主机节点
Request createHostNodeRequest = RequestBuilder.newBuilder()
.method("graph.update")
.paramEntry("graphid", graphId)
.paramEntry("gitems", new GraphItem[]{
new GraphItem()
.color("00AA00")
.itemid("12345")
.calc_fnc("AVERAGE")
.type(GraphType.ITEM_GRAPH)
.graphid(graphId)
.x(100)
.y(100)
.width(100)
.height(100)
.hostid("67890")
.name("My Host")
})
.build();
zabbixApi.call(createHostNodeRequest);
// 创建设备节点
Request createDeviceNodeRequest = RequestBuilder.newBuilder()
.method("graph.update")
.paramEntry("graphid", graphId)
.paramEntry("gitems", new GraphItem[]{
new GraphItem()
.color("AA0000")
.itemid("67890")
.calc_fnc("AVERAGE")
.type(GraphType.ITEM_GRAPH)
.graphid(graphId)
.x(300)
.y(300)
.width(100)
.height(100)
.name("My Device")
})
.build();
zabbixApi.call(createDeviceNodeRequest);
// 创建连线
Request createLineRequest = RequestBuilder.newBuilder()
.method("graph.update")
.paramEntry("graphid", graphId)
.paramEntry("gitems", new GraphItem[]{
new GraphItem()
.color("0000AA")
.type(GraphType.LINE)
.graphid(graphId)
.x(150)
.y(150)
.width(300)
.height(300)
.itemid("0")
.drawtype(0)
.from_hostid("67890")
.to_hostid("0")
})
.build();
zabbixApi.call(createLineRequest);
// 获取拓扑图信息
Request getGraphRequest = RequestBuilder.newBuilder()
.method("graph.get")
.paramEntry("graphids", graphId)
.build();
System.out.println(zabbixApi.call(getGraphRequest).getResult()); // 输出拓扑图信息
// 关闭 Zabbix API 连接
zabbixApi.logout();
}
}
```
需要注意的是,这只是一个简单的示例,实际上创建拓扑图需要考虑更多的因素,例如节点和连线之间的排列、节点和连线的样式等。您可以根据自己的需求进行修改和扩展。
相关推荐
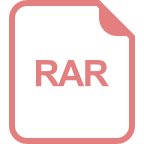
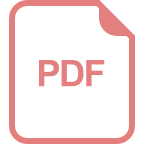














