用c语言写一个贪吃蛇游戏”的说明如下:(参照演示程序和源程序框架)启动程序时,输入的用户名如果以前没有,则系统自动完成注册,如果有,则进行密码比较以判断是否允许登录。用户名将用于英雄榜中。
时间: 2024-03-19 09:45:39 浏览: 12
好的,这是一个比较复杂的问题。首先,你需要了解贪吃蛇游戏的规则和逻辑,然后你需要学习如何使用C语言进行编程。
以下是一个简单的贪吃蛇游戏的源程序框架,可以帮助你开始编写你自己的贪吃蛇游戏:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define MAX_X 20
#define MAX_Y 20
enum direction {UP, DOWN, LEFT, RIGHT};
struct snake {
int x;
int y;
struct snake *next;
};
struct snake *head, *tail;
int food_x, food_y;
enum direction dir = RIGHT;
int score = 0;
char username[20];
void init_game();
void init_snake();
void init_food();
void display();
void update();
void game_over();
void save_score();
int check_collision(int x, int y);
void register_user();
void login();
int main() {
int choice;
printf("Welcome to Snake Game!\n");
printf("1. Register\n");
printf("2. Login\n");
printf("Enter your choice: ");
scanf("%d", &choice);
if (choice == 1) {
register_user();
} else if (choice == 2) {
login();
} else {
printf("Invalid choice!\n");
exit(0);
}
init_game();
while (1) {
display();
update();
Sleep(100);
}
return 0;
}
void init_game() {
srand(time(NULL));
init_snake();
init_food();
}
void init_snake() {
head = (struct snake*) malloc(sizeof(struct snake));
tail = (struct snake*) malloc(sizeof(struct snake));
head->x = 3;
head->y = 1;
head->next = tail;
tail->x = 1;
tail->y = 1;
tail->next = NULL;
}
void init_food() {
food_x = rand() % MAX_X + 1;
food_y = rand() % MAX_Y + 1;
}
void display() {
system("cls");
printf("Score: %d\n", score);
for (int i = 0; i < MAX_X; i++) {
for (int j = 0; j < MAX_Y; j++) {
if (i == head->x && j == head->y) {
printf("O");
} else if (i == food_x && j == food_y) {
printf("*");
} else {
int found = 0;
struct snake *ptr = head->next;
while (ptr != tail) {
if (ptr->x == i && ptr->y == j) {
printf("o");
found = 1;
break;
}
ptr = ptr->next;
}
if (!found) {
printf(" ");
}
}
}
printf("\n");
}
}
void update() {
int new_x = head->x, new_y = head->y;
switch (dir) {
case UP:
new_x--;
break;
case DOWN:
new_x++;
break;
case LEFT:
new_y--;
break;
case RIGHT:
new_y++;
break;
}
if (check_collision(new_x, new_y)) {
game_over();
return;
}
struct snake *new_head = (struct snake*) malloc(sizeof(struct snake));
new_head->x = new_x;
new_head->y = new_y;
new_head->next = head;
head = new_head;
if (new_x == food_x && new_y == food_y) {
score++;
init_food();
} else {
struct snake *ptr = head;
while (ptr->next != tail) {
ptr = ptr->next;
}
struct snake *old_tail = tail;
tail = ptr;
free(old_tail);
}
}
void game_over() {
printf("Game over! Your score is %d.\n", score);
save_score();
exit(0);
}
void save_score() {
FILE *fp;
fp = fopen("high_scores.txt", "a");
fprintf(fp, "%s %d\n", username, score);
fclose(fp);
}
int check_collision(int x, int y) {
if (x < 0 || x >= MAX_X || y < 0 || y >= MAX_Y) {
return 1;
}
struct snake *ptr = head->next;
while (ptr != tail) {
if (ptr->x == x && ptr->y == y) {
return 1;
}
ptr = ptr->next;
}
return 0;
}
void register_user() {
char password[20], confirm[20];
FILE *fp;
fp = fopen("users.txt", "a+");
printf("Enter your username: ");
scanf("%s", username);
while (!feof(fp)) {
char name[20], pass[20];
fscanf(fp, "%s %s", name, pass);
if (strcmp(username, name) == 0) {
printf("Username already exists!\n");
fclose(fp);
exit(0);
}
}
printf("Enter your password: ");
scanf("%s", password);
printf("Confirm your password: ");
scanf("%s", confirm);
if (strcmp(password, confirm) != 0) {
printf("Passwords do not match!\n");
fclose(fp);
exit(0);
}
fprintf(fp, "%s %s\n", username, password);
fclose(fp);
}
void login() {
char password[20];
FILE *fp;
fp = fopen("users.txt", "r");
printf("Enter your username: ");
scanf("%s", username);
while (!feof(fp)) {
char name[20], pass[20];
fscanf(fp, "%s %s", name, pass);
if (strcmp(username, name) == 0) {
printf("Enter your password: ");
scanf("%s", password);
if (strcmp(password, pass) != 0) {
printf("Incorrect password!\n");
fclose(fp);
exit(0);
}
fclose(fp);
return;
}
}
printf("Username does not exist!\n");
fclose(fp);
exit(0);
}
```
这个程序包含了很多功能,包括注册、登录、保存分数、检查碰撞等等。你可以按照程序框架,编写自己的贪吃蛇游戏程序。
相关推荐
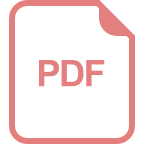
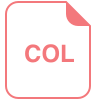













