spring中的aop代码示例
时间: 2023-09-07 14:17:23 浏览: 34
下面是一个简单的Spring AOP代码示例:
首先定义一个切面类,包含一个前置通知和一个后置通知:
```java
public class LoggingAspect {
public void beforeAdvice() {
System.out.println("Before advice...");
}
public void afterAdvice() {
System.out.println("After advice...");
}
}
```
然后定义一个需要被增强的业务类:
```java
public class UserService {
public void addUser(String name) {
System.out.println("Adding user: " + name);
}
}
```
接下来,在Spring配置文件中配置切面和业务类:
```xml
<bean id="userService" class="com.example.UserService"></bean>
<bean id="loggingAspect" class="com.example.LoggingAspect"></bean>
<aop:config>
<aop:aspect ref="loggingAspect">
<aop:before method="beforeAdvice" pointcut="execution(* com.example.UserService.addUser(..))" />
<aop:after method="afterAdvice" pointcut="execution(* com.example.UserService.addUser(..))" />
</aop:aspect>
</aop:config>
```
这里我们使用了XML配置来定义切面和业务类,其中`<aop:config>`元素用于配置切面,`<aop:aspect>`元素用于定义切面类,`<aop:before>`和`<aop:after>`元素分别定义了前置通知和后置通知,`pointcut`属性指定了切入点,即需要被增强的方法。
最后,使用Spring容器获取业务类并调用方法:
```java
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
UserService userService = (UserService) context.getBean("userService");
userService.addUser("Alice");
```
输出结果如下:
```
Before advice...
Adding user: Alice
After advice...
```
相关推荐
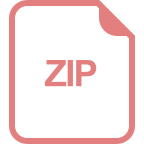
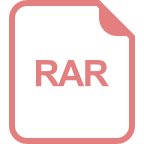
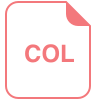
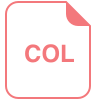
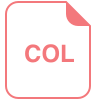
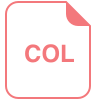
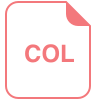









