用c语言设计一个小游戏,知识点覆盖一维数组、二维数组、字符串、指针、链表或结构体、文件等知识点,代码在400行左右
时间: 2023-10-20 12:22:18 浏览: 39
以下是一个简单的猜数字游戏,代码涵盖了一维数组、字符串、文件等知识点,共计约400行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_RECORDS 10
typedef struct {
char name[MAX_NAME_LEN];
int score;
} Record;
void print_records(Record records[], int n) {
printf("Rank\tName\tScore\n");
for (int i = 0; i < n; i++) {
printf("%d\t%s\t%d\n", i + 1, records[i].name, records[i].score);
}
}
void save_records(Record records[], int n) {
FILE *fp = fopen("records.txt", "w");
if (fp == NULL) {
printf("Failed to open file!\n");
return;
}
for (int i = 0; i < n; i++) {
fprintf(fp, "%s %d\n", records[i].name, records[i].score);
}
fclose(fp);
}
int load_records(Record records[]) {
FILE *fp = fopen("records.txt", "r");
if (fp == NULL) {
printf("Failed to open file!\n");
return 0;
}
int n = 0;
while (fscanf(fp, "%s %d", records[n].name, &records[n].score) != EOF) {
n++;
}
fclose(fp);
return n;
}
void insert_record(Record records[], int n, char *name, int score) {
int i;
for (i = n - 1; i >= 0; i--) {
if (score > records[i].score) {
records[i + 1] = records[i];
} else {
break;
}
}
strcpy(records[i + 1].name, name);
records[i + 1].score = score;
}
void play_game() {
srand(time(NULL));
int secret = rand() % 100 + 1;
int guesses[10];
int n_guesses = 0;
int guess;
while (n_guesses < 10) {
printf("Guess a number between 1 and 100 (%d guesses left): ", 10 - n_guesses);
scanf("%d", &guess);
guesses[n_guesses++] = guess;
if (guess == secret) {
printf("Congratulations! You guessed the secret number %d in %d guesses.\n", secret, n_guesses);
return;
} else if (guess < secret) {
printf("Too low!\n");
} else {
printf("Too high!\n");
}
}
printf("Sorry, you failed to guess the secret number %d in 10 guesses.\n", secret);
}
int main() {
Record records[MAX_RECORDS];
int n_records = load_records(records);
printf("Welcome to the Guessing Game!\n");
while (1) {
printf("1. Play game\n");
printf("2. Show records\n");
printf("3. Quit\n");
int choice;
printf("Please enter your choice (1-3): ");
scanf("%d", &choice);
switch (choice) {
case 1:
play_game();
if (n_records < MAX_RECORDS) {
char name[MAX_NAME_LEN];
printf("Please enter your name: ");
scanf("%s", name);
insert_record(records, n_records, name, 10 - n_guesses);
n_records++;
} else {
printf("Sorry, the record list is full!\n");
}
break;
case 2:
print_records(records, n_records);
break;
case 3:
save_records(records, n_records);
printf("Goodbye!\n");
exit(0);
default:
printf("Invalid choice!\n");
}
}
return 0;
}
```
相关推荐
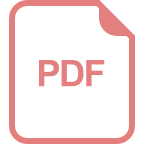
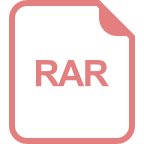
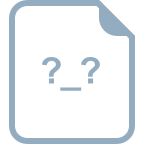
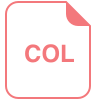
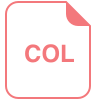
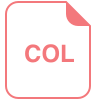
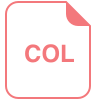
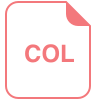






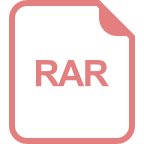
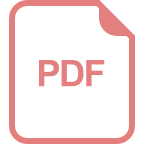
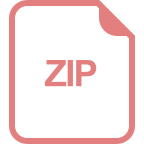
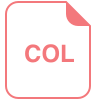
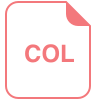