.netmvc 图片插入mysql
时间: 2023-07-08 16:53:07 浏览: 111
将图片插入 MySQL 数据库中,可以将图片转换成 Base64 编码的字符串,然后将该字符串插入到数据库中的相应字段中。
下面是一个使用 .NET MVC 框架实现将图片插入 MySQL 数据库的示例代码:
1. 首先,创建一个包含图片上传表单的视图文件,如下所示:
```
@using (Html.BeginForm("Upload", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<input type="file" name="file" />
<input type="submit" value="Upload" />
}
```
2. 接着,在 HomeController 中创建一个用于处理上传图片的方法,如下所示:
```
[HttpPost]
public ActionResult Upload(HttpPostedFileBase file)
{
if (file != null && file.ContentLength > 0)
{
byte[] bytes = new byte[file.ContentLength];
file.InputStream.Read(bytes, 0, file.ContentLength);
string base64String = Convert.ToBase64String(bytes);
// 将 base64String 插入到数据库中相应字段中
// ...
}
return RedirectToAction("Index");
}
```
3. 在 MySQL 数据库中创建一个表,用于存储图片信息,包括图片的名称、类型和 Base64 编码字符串,如下所示:
```
CREATE TABLE `images` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) DEFAULT NULL,
`type` varchar(255) DEFAULT NULL,
`data` longtext,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
4. 在 Upload 方法中,使用 ADO.NET 连接到 MySQL 数据库,并将 Base64 编码字符串插入到数据库中相应字段中,如下所示:
```
string connectionString = "Server=localhost;Database=test;Uid=root;Pwd=123456;";
using (MySqlConnection connection = new MySqlConnection(connectionString))
{
string query = "INSERT INTO images (name, type, data) VALUES (@name, @type, @data)";
using (MySqlCommand command = new MySqlCommand(query, connection))
{
command.Parameters.AddWithValue("@name", file.FileName);
command.Parameters.AddWithValue("@type", file.ContentType);
command.Parameters.AddWithValue("@data", base64String);
connection.Open();
command.ExecuteNonQuery();
}
}
```
这样,就可以将图片插入 MySQL 数据库中了。当需要获取图片时,可以从数据库中读取 Base64 编码字符串,并将其转换成图片,如下所示:
```
public ActionResult GetImage(int id)
{
byte[] bytes = null;
string base64String = null;
string contentType = null;
string connectionString = "Server=localhost;Database=test;Uid=root;Pwd=123456;";
using (MySqlConnection connection = new MySqlConnection(connectionString))
{
string query = "SELECT type, data FROM images WHERE id = @id";
using (MySqlCommand command = new MySqlCommand(query, connection))
{
command.Parameters.AddWithValue("@id", id);
connection.Open();
using (MySqlDataReader reader = command.ExecuteReader())
{
if (reader.Read())
{
contentType = reader.GetString(0);
base64String = reader.GetString(1);
bytes = Convert.FromBase64String(base64String);
}
}
}
}
return File(bytes, contentType);
}
```
在上述代码中,GetImage 方法根据图片的 ID 从数据库中读取相应的 Base64 编码字符串,并将其转换成字节数组。最后,将字节数组和图片类型作为参数传递给 File 方法,返回图片。
阅读全文
相关推荐



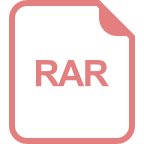






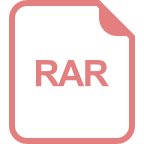







