c++使用hdf5读取python使用h5py存储的mat文件的变量时,变量的类型为Unkown
时间: 2024-02-17 19:02:06 浏览: 151
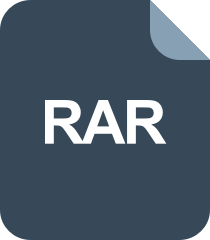
hdf5 for C++
这个问题可能是由于C++程序没有正确解析h5py存储的mat文件导致的。h5py是一种Python库,用于读写HDF5文件,并且支持Python中的Numpy数组。因此,如果你想在C++中读取由h5py存储的Numpy数组,你需要使用HDF5 C++库,并且需要了解Numpy数组在HDF5文件中的存储结构。
在C++中,你需要使用HDF5 C++库中的H5File类打开HDF5文件,并使用H5DataSet类读取数据集。然后你可以使用read()方法从数据集中读取数据,并将其存储到一个C++变量中。需要注意的是,HDF5 C++库中的数据类型与Numpy数据类型不完全相同,因此你需要使用H5T类来转换数据类型。
总体来说,你需要了解以下几个步骤:
1. 使用H5File类打开HDF5文件
2. 使用H5DataSet类读取数据集
3. 使用H5T类转换数据类型(如果需要)
4. 使用read()方法读取数据,并将其存储到C++变量中
下面是一个简单的示例代码,可以帮助你开始使用HDF5 C++库读取HDF5文件中的数据:
```c++
#include <iostream>
#include <string>
#include "H5Cpp.h"
// Define the name of the file and the dataset
const H5std_string FILE_NAME("example.h5");
const H5std_string DATASET_NAME("my_dataset");
int main() {
try {
// Open the file and the dataset
H5::H5File file(FILE_NAME, H5F_ACC_RDONLY);
H5::DataSet dataset = file.openDataSet(DATASET_NAME);
// Get the data type of the dataset
H5::DataType datatype = dataset.getDataType();
// Get the dataspace of the dataset
H5::DataSpace dataspace = dataset.getSpace();
// Get the number of dimensions in the dataset
int rank = dataspace.getSimpleExtentNdims();
// Get the dimensions of the dataset
hsize_t dims[rank];
dataspace.getSimpleExtentDims(dims, NULL);
// Create a buffer to store the data
int data[dims[0]][dims[1]];
// Read the data from the dataset
dataset.read(data, datatype);
// Print the data to the console
for (int i = 0; i < dims[0]; i++) {
for (int j = 0; j < dims[1]; j++) {
std::cout << data[i][j] << " ";
}
std::cout << std::endl;
}
// Close the dataset and the file
dataset.close();
file.close();
}
catch (H5::Exception& e) {
std::cerr << "Error reading HDF5 file: " << e.getCDetailMsg() << std::endl;
return -1;
}
return 0;
}
```
需要注意的是,在上面的示例代码中,我们假设数据集的数据类型为int,数据集的维度为2。如果你的数据集的数据类型和维度不同,你需要相应地修改代码。
阅读全文
相关推荐
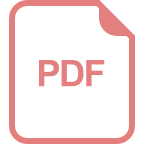














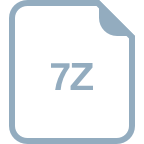