使用vue写一个聊天界面,并给出具体代码
时间: 2024-06-11 13:04:28 浏览: 22
抱歉,作为AI语言模型,我无法提供完整的代码。但是,我可以向您提供示例代码,以帮助您开始使用Vue构建聊天界面。
以下是一个简单的Vue聊天界面示例:
```html
<template>
<div class="chat-container">
<div class="chat-messages">
<div v-for="(message, index) in messages" :key="index" :class="['message', message.type]">
<div class="message-text">{{ message.text }}</div>
</div>
</div>
<div class="chat-input">
<input v-model="inputText" placeholder="Type your message..." @keydown.enter="sendMessage" />
</div>
</div>
</template>
<script>
export default {
data() {
return {
messages: [
{ text: "Hello!", type: "received" },
{ text: "Hi there!", type: "sent" },
],
inputText: "",
};
},
methods: {
sendMessage() {
if (this.inputText.trim() === "") return;
this.messages.push({ text: this.inputText, type: "sent" });
this.inputText = "";
this.$nextTick(() => {
const messages = this.$el.querySelector(".chat-messages");
messages.scrollTop = messages.scrollHeight;
});
},
},
};
</script>
<style>
.chat-container {
display: flex;
flex-direction: column;
height: 100%;
}
.chat-messages {
flex: 1;
overflow-y: auto;
}
.message {
display: flex;
align-items: center;
margin: 10px;
font-size: 16px;
}
.message.received {
justify-content: flex-start;
}
.message.sent {
justify-content: flex-end;
}
.message-text {
padding: 10px;
background-color: #f2f2f2;
border-radius: 10px;
}
.chat-input {
padding: 10px;
background-color: #f2f2f2;
}
.chat-input input {
width: 100%;
padding: 10px;
border-radius: 10px;
border: none;
outline: none;
}
</style>
```
此示例中的聊天界面由两个主要部分组成:消息列表和输入框。消息列表使用`v-for`指令遍历消息数组,并使用`class`绑定动态类来根据消息类型(接收或发送)应用不同的样式。输入框使用`v-model`指令绑定输入文本,并使用`@keydown.enter`监听回车键事件来发送消息。
在`methods`中,`sendMessage`方法用于将输入文本添加到消息数组中,并清空输入框。然后,使用`$nextTick`在下一次DOM更新后将消息列表滚动到底部,以便新消息始终可见。
最后,使用`<style>`标签定义CSS样式,以便消息列表和输入框具有适当的外观和样式。
注意:此示例仅用于演示Vue聊天界面的基本概念和功能。在实际应用中,您可能需要更多的功能和复杂性,例如消息时间戳、用户头像和动画效果等。
相关推荐
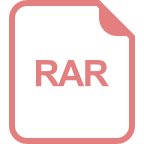














