举例ant design 用call方法,dispatch,fetch,axios进行前后端数据交互的例子
时间: 2024-05-12 12:14:33 浏览: 111
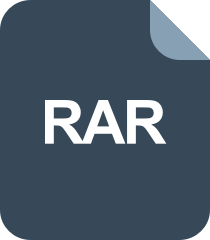
ant design 组件案例
Ant Design 是一个 UI 组件库,它并不涉及具体的数据交互方式。因此,以下是使用 React、Redux 和 Axios 进行前后端数据交互的例子。
假设我们有一个需求,需要从后端获取用户列表并展示在前端页面上。以下是实现这个需求的步骤:
1. 创建 Redux Store
首先,在 Redux 中创建一个 store,用于管理应用的状态。
```js
import { createStore, applyMiddleware } from 'redux';
import thunkMiddleware from 'redux-thunk';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(thunkMiddleware)
);
export default store;
```
2. 创建 Redux Action
接下来,创建一个 Redux action,用于从后端获取用户列表数据。
```js
import axios from 'axios';
export const fetchUsers = () => {
return dispatch => {
dispatch({ type: 'FETCH_USERS_REQUEST' });
axios.get('/api/users')
.then(response => {
dispatch({
type: 'FETCH_USERS_SUCCESS',
payload: response.data
});
})
.catch(error => {
dispatch({
type: 'FETCH_USERS_FAILURE',
payload: error
});
});
};
};
```
3. 创建 Redux Reducer
然后,创建一个 Redux reducer,用于处理用户列表数据的状态。
```js
const initialState = {
users: [],
isLoading: false,
error: null
};
const userReducer = (state = initialState, action) => {
switch (action.type) {
case 'FETCH_USERS_REQUEST':
return {
...state,
isLoading: true,
error: null
};
case 'FETCH_USERS_SUCCESS':
return {
...state,
isLoading: false,
users: action.payload
};
case 'FETCH_USERS_FAILURE':
return {
...state,
isLoading: false,
error: action.payload
};
default:
return state;
}
};
export default userReducer;
```
4. 在组件中使用数据
最后,在 React 组件中使用上述创建的 Redux store 和 action。
```js
import React, { useEffect } from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { fetchUsers } from './actions';
const UserList = () => {
const dispatch = useDispatch();
const users = useSelector(state => state.users);
const isLoading = useSelector(state => state.isLoading);
const error = useSelector(state => state.error);
useEffect(() => {
dispatch(fetchUsers());
}, [dispatch]);
if (isLoading) {
return <div>Loading...</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
};
export default UserList;
```
这样,当用户访问 `UserList` 组件时,会触发 `fetchUsers` action,从后端获取用户列表数据并更新 Redux store 中的状态。然后,组件会根据 Redux store 中的状态展示用户列表或错误信息或加载中状态。
阅读全文
相关推荐
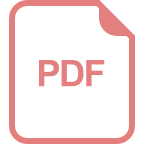

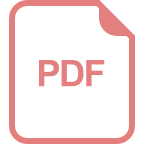
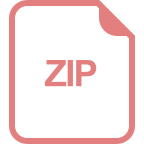
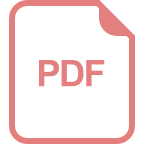
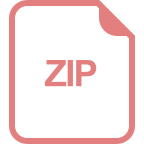
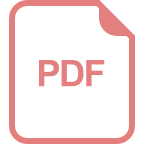
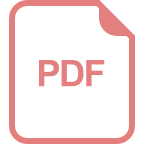
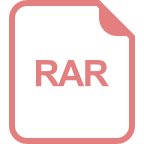
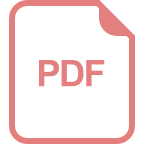
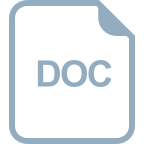
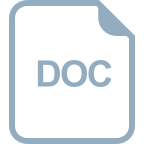
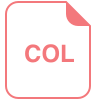
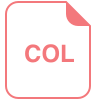
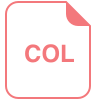

