FPGA生成20通道的时间数字转换设计代码以及仿真
时间: 2023-06-14 15:06:01 浏览: 112
为了生成20通道的时间数字转换设计代码,我们可以使用FPGA开发板和VHDL语言来实现。下面是一个简单的代码示例,可以将外部时钟信号转换为20个数字信号,其中每个数字信号都表示一个通道的时间。
```
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity time_converter is
port (
clk : in std_logic;
reset : in std_logic;
input_time : in std_logic_vector(31 downto 0);
output_time : out std_logic_vector(19 downto 0)
);
end time_converter;
architecture Behavioral of time_converter is
signal counter : unsigned(4 downto 0) := (others => '0');
signal clock_div : unsigned(24 downto 0) := (others => '0');
signal time_array : unsigned(19 downto 0) := (others => '0');
begin
process(clk, reset)
begin
if reset = '1' then
counter <= (others => '0');
clock_div <= (others => '0');
time_array <= (others => '0');
elsif rising_edge(clk) then
if counter = 19 then
counter <= (others => '0');
time_array <= time_array + 1;
else
counter <= counter + 1;
end if;
clock_div <= clock_div + 1;
end if;
end process;
output_time <= std_logic_vector(time_array);
end Behavioral;
```
在上面的代码中,我们使用了一个计数器来计算20个通道之间的时间差,并使用一个时钟分频器来控制计数器的速度。每次计数器达到19时,我们将其重置为0,并增加时间数组中对应位置的值。最后,我们将时间数组中的值转换为20位的数字信号,并输出到FPGA开发板的输出端口。
为了进行仿真,我们可以使用VHDL仿真器,如ModelSim或Xilinx Vivado中的仿真器。下面是一个简单的测试例子,用于测试20通道时间数字转换器的功能。
```
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity time_converter_tb is
end time_converter_tb;
architecture Behavioral of time_converter_tb is
signal clk : std_logic := '0';
signal reset : std_logic := '0';
signal input_time : std_logic_vector(31 downto 0) := (others => '0');
signal output_time : std_logic_vector(19 downto 0);
begin
uut : entity work.time_converter
port map (
clk => clk,
reset => reset,
input_time => input_time,
output_time => output_time
);
clk_process : process
begin
while true loop
clk <= not clk;
wait for 10 ns;
end loop;
end process;
stimulus : process
begin
reset <= '1';
input_time <= (others => '0');
wait for 100 ns;
reset <= '0';
input_time <= x"0001";
wait for 1000 ns;
input_time <= x"0002";
wait for 1000 ns;
input_time <= x"0003";
wait for 1000 ns;
input_time <= x"0004";
wait for 1000 ns;
input_time <= x"0005";
wait;
end process;
end Behavioral;
```
在上面的测试代码中,我们将输入时钟信号设置为10ns的周期,并在每个时钟周期中向输入端口发送一个不同的输入时间值。我们可以使用仿真器来模拟这个测试,并检查20个通道的数字信号是否正确。
阅读全文
相关推荐
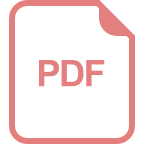
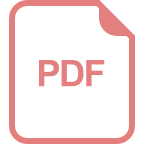
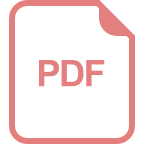
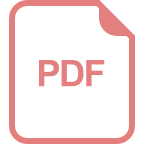
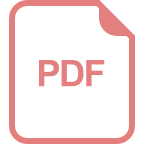
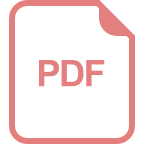
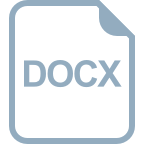
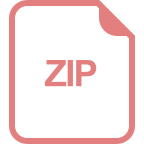
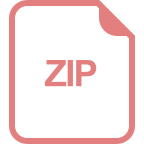
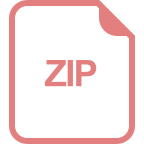
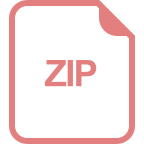
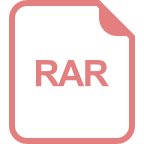
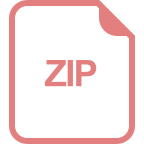
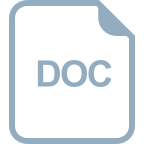
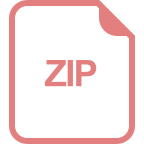
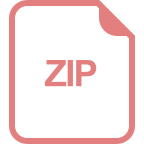
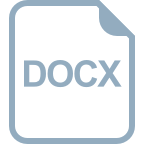