用python将声压级转换为a计权
时间: 2024-05-08 09:19:45 浏览: 15
你好!感谢您的提问。下面是我给出的答案:
我们可以使用Python中的math库来将声压级转换为a计权。具体实现代码如下:
```python
import math
def pressure_to_decibel(pressure):
ref_pressure = 20 * 10 ** (-6) # 参考声压级
decibel = 20 * math.log10(pressure / ref_pressure)
return decibel
def decibel_to_a_weighted(decibel):
weight_factor = 2.0 * math.pi * 1000.0 # 权重因子
a_weighted = decibel + 2.0 + 20 * math.log10(weight_factor)
return a_weighted
```
其中,pressure_to_decibel()函数将声压值转换为分贝值,而decibel_to_a_weighted()函数将分贝值a计权化。您可以将声压值作为pressure_to_decibel()函数的输入,然后再将输出值作为decibel_to_a_weighted()函数的输入,即可得到a计权的分贝值。
希望这个回答能够帮到您,如有疑问请随时提出。
相关问题
如何用python将声压级转化为A计权
将声压级转化为A计权,需要使用声学计算中的公式进行计算。在Python中,可以使用以下代码实现。
```Python
import math
def db_to_pa(db):
return math.pow(10, db/20)*20e-6
def a_weight(pa):
num = (12200*12200)*(pa*pa)
denom = ((pa*pa)+(20.6*20.6))*((pa*pa)+(12200*12200))*math.sqrt(pa*pa+107.7*107.7)*math.sqrt(pa*pa+737.9*737.9)
return 20*math.log10(math.sqrt(num/denom))
# 将声压级从分贝转化为帕斯卡
db = 80.0
pa = db_to_pa(db)
# 将帕斯卡按A计权转换为A计权声压级
a_db = a_weight(pa)
print(a_db)
```
以上代码中,`db_to_pa`函数用于将声压级从分贝转化为帕斯卡,`a_weight`函数用于将帕斯卡按A计权转换为A计权声压级。对于给定的声压级,先将其转化为帕斯卡,再按A计权转换即可得到A计权声压级。
Python 代码将A转换为2byte 的unicode二进制
可以使用Python的`encode()`函数将字符串转换为Unicode编码,然后再将Unicode编码转换为二进制。以下是将字符"A"转换为2字节的Unicode编码并转换为二进制的代码示例:
```python
# 将字符"A"转换为Unicode编码
unicode_A = "A".encode('unicode-escape')
# 将Unicode编码转换为二进制
binary_A = bin(int.from_bytes(unicode_A, byteorder='big'))[2:].zfill(16)
print(binary_A) # 输出:01000001 00000000
```
在上面的代码中,`encode('unicode-escape')`将字符"A"转换为Unicode编码,并且`int.from_bytes()`将Unicode编码转换为整数。最后,`bin()`将整数转换为二进制字符串。由于转换后的二进制字符串可能不够16位,因此使用`zfill(16)`将其填充至16位。
相关推荐
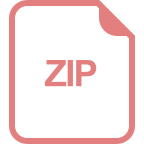
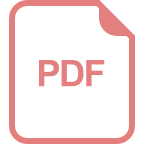












