修改版本1中Fan类,让其继承Pane类,并且把color属性设置为Color类型,默认属性为red。随机产生radius,取值范围为1-5;随机产生颜色,取值范围为red、blue、yellow、green、orange;根据color、radius属性值绘制风扇并且写出main函数
时间: 2024-03-30 22:39:52 浏览: 26
好的,根据您的要求,我为您修改了Fan类并且提供了一个包含main函数的测试类。以下是修改后的代码:
Fan.java
```java
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Arc;
import javafx.scene.shape.ArcType;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Polygon;
public class Fan extends Pane {
private Color color;
private int radius;
public Fan() {
this.color = Color.RED;
this.radius = (int) (Math.random() * 5) + 1;
draw();
}
public Fan(Color color, int radius) {
this.color = color;
this.radius = radius;
draw();
}
public void setColor(Color color) {
this.color = color;
draw();
}
public void setRadius(int radius) {
this.radius = radius;
draw();
}
private void draw() {
// 清空面板
getChildren().clear();
// 绘制风扇
Circle circle = new Circle(50, 50, 50);
circle.setFill(Color.WHITE);
circle.setStroke(Color.BLACK);
getChildren().add(circle);
double startAngle = 15;
double angleStep = 90;
for (int i = 0; i < 4; i++) {
Arc arc = new Arc(50, 50, 40, 40, startAngle, 30);
arc.setFill(color);
arc.setType(ArcType.ROUND);
getChildren().add(arc);
// 绘制扇叶
double x1 = 50 + 40 * Math.cos(Math.toRadians(startAngle));
double y1 = 50 + 40 * Math.sin(Math.toRadians(startAngle));
double x2 = 50 + 40 * Math.cos(Math.toRadians(startAngle + angleStep / 2));
double y2 = 50 + 40 * Math.sin(Math.toRadians(startAngle + angleStep / 2));
double x3 = 50 + (40 + radius * 10) * Math.cos(Math.toRadians(startAngle + angleStep / 4));
double y3 = 50 + (40 + radius * 10) * Math.sin(Math.toRadians(startAngle + angleStep / 4));
double x4 = 50 + (40 + radius * 10) * Math.cos(Math.toRadians(startAngle + angleStep * 3 / 4));
double y4 = 50 + (40 + radius * 10) * Math.sin(Math.toRadians(startAngle + angleStep * 3 / 4));
Polygon polygon = new Polygon(x1, y1, x3, y3, x2, y2, x4, y4);
polygon.setFill(color);
getChildren().add(polygon);
startAngle += angleStep;
}
}
}
```
FanTest.java
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.HBox;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class FanTest extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
// 创建3个风扇对象
Fan fan1 = new Fan();
Fan fan2 = new Fan(Color.BLUE, 3);
Fan fan3 = new Fan(Color.ORANGE, 5);
// 将3个风扇对象添加到HBox面板中
HBox root = new HBox(fan1, fan2, fan3);
// 创建场景并显示
Scene scene = new Scene(root, 300, 100);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在这个版本中,Fan类继承了Pane类,并且新增了color和radius属性。在默认构造方法中,我们随机生成了radius和color值,然后调用draw()方法绘制风扇。在draw()方法中,我们首先清空面板,然后绘制圆形和4个扇形。接着,我们计算出扇形对应的扇叶的4个点的坐标,绘制出扇叶。最后,我们将圆形、扇形、扇叶添加到面板上。
测试类FanTest中,我们创建了3个风扇对象,其中一个使用默认构造方法,另外两个使用指定颜色和半径参数的构造方法。然后,我们将3个风扇对象添加到HBox面板中,并将HBox面板添加到场景中,最后显示场景。
希望这个修改后的Fan类和测试类能够满足您的需求,如果您有其他问题或者需要进一步的帮助,请随时提问!
相关推荐
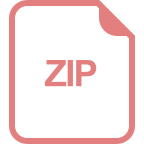
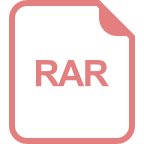
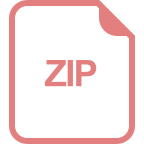


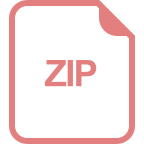
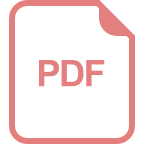
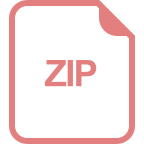
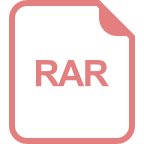
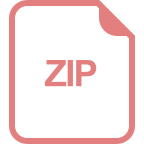
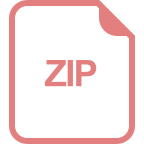
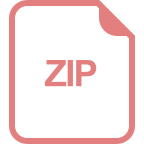