用js es5的方法写一个class
时间: 2024-01-28 11:03:26 浏览: 73
好的,以下是用 ES5 的方法写一个类的例子:
```javascript
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.sayHello = function() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
const john = new Person('John', 25);
john.sayHello(); // Output: Hello, my name is John and I am 25 years old.
```
这个例子中,我们定义了一个名为 `Person` 的类,它有两个属性 `name` 和 `age`,以及一个方法 `sayHello`。我们使用 `this` 来访问类的属性,使用 `prototype` 来添加方法。最后,我们使用 `new` 运算符创建了一个 `Person` 实例,并调用了 `sayHello` 方法。
相关问题
复杂的ES5函数转化ES6的class
将一个复杂的ES5函数转换为ES6类的步骤如下:
1. 创建一个类名,用于替代函数名。
2. 将函数体中的属性和方法移动到类的构造函数中,并使用“this”关键字引用它们。
3. 将函数体中的“prototype”属性中的所有方法转移到类定义中作为类方法。
4. 删除原函数定义。
下面是一个示例代码:
ES5函数:
```javascript
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.getName = function() {
return this.name;
};
Person.prototype.getAge = function() {
return this.age;
};
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`);
};
```
转换为ES6类:
```javascript
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
getName() {
return this.name;
}
getAge() {
return this.age;
}
greet() {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`);
}
}
```
超级复杂的ES5函数转化ES6的class
将一个超级复杂的 ES5 函数转化为 ES6 的类可能需要花费一些时间和精力,但是可以按照下面的步骤来实现:
1. 创建一个类,并在其构造函数中设置所有的属性和方法。
2. 将原始函数中的所有属性和方法移动到类的原型中。
3. 将原始函数中的所有静态属性和方法移动到类本身。
下面是一个示例,将一个超级复杂的 ES5 函数转化为 ES6 的类:
```javascript
function SuperComplexFunction(name) {
this.name = name;
}
SuperComplexFunction.prototype.sayHello = function() {
console.log("Hello, " + this.name);
};
SuperComplexFunction.staticMethod = function() {
console.log("This is a static method");
};
class SuperComplexClass {
constructor(name) {
this.name = name;
}
sayHello() {
console.log("Hello, " + this.name);
}
static staticMethod() {
console.log("This is a static method");
}
}
Object.assign(SuperComplexClass, {staticMethod: SuperComplexFunction.staticMethod});
// Usage
const complexInstance = new SuperComplexClass("World");
complexInstance.sayHello(); // Hello, World
SuperComplexClass.staticMethod(); // This is a static method
```
在上面的示例中,我们将原始函数 SuperComplexFunction 转化为了 ES6 的类 SuperComplexClass。我们首先创建了一个构造函数,并将原始函数中的属性和方法移动到了构造函数中。然后,我们将原始函数中的原型属性和方法移动到类的原型中。最后,我们将原始函数中的静态属性和方法移动到了类本身。
阅读全文
相关推荐
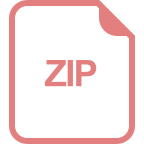
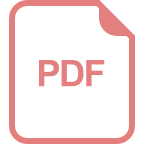
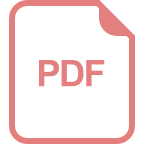
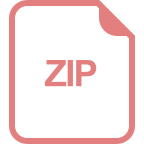
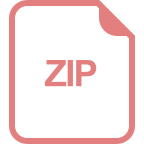
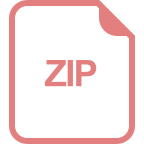
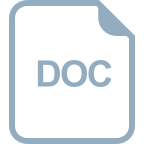
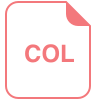
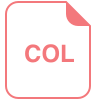
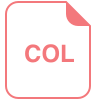
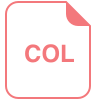
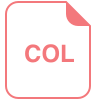


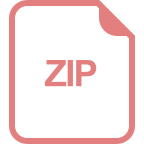
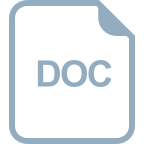