import FreeCAD import FreeCADGui from PySide import QtGui, QtCore Gui.activateWorkbench("PartWorkbench") FreeCAD.newDocument() class SphereDialog(QtGui.QDialog): def __init__(self): super().__init__() self.setWindowTitle("Create Sphere") self.create_widgets() def create_widgets(self): layout = QtGui.QVBoxLayout() # Radius widget radius_label = QtGui.QLabel("Radius:") self.radius_spinbox = QtGui.QDoubleSpinBox() self.radius_spinbox.setMinimum(0.1) self.radius_spinbox.setMaximum(1000.0) self.radius_spinbox.setValue(10.0) layout.addWidget(radius_label) layout.addWidget(self.radius_spinbox) # Opacity widget opacity_label = QtGui.QLabel("Opacity:") self.opacity_slider = QtGui.QSlider(QtCore.Qt.Horizontal) self.opacity_slider.setMinimum(0) self.opacity_slider.setMaximum(100) self.opacity_slider.setValue(50) layout.addWidget(opacity_label) layout.addWidget(self.opacity_slider) # Create button create_button = QtGui.QPushButton("Create") create_button.clicked.connect(self.create_sphere) layout.addWidget(create_button) self.setLayout(layout) def create_sphere(self): # Get sphere parameters radius = self.radius_spinbox.value() opacity = float(self.opacity_slider.value() / 100) # Create sphere sphere = FreeCAD.ActiveDocument.addObject("Part::Sphere", "Sphere") sphere.Radius = radius sphere.ViewObject.Transparency = int(round(opacity * 100)) # Show in 3D view sphere.ViewObject.Visibility = True FreeCADGui.SendMsgToActiveView("ViewFit") FreeCADGui.Selection.clearSelection() FreeCADGui.Selection.addSelection(sphere) FreeCADGui.SendMsgToActiveView("ViewFit") # Close dialog self.close() dialog = SphereDialog() dialog.show()代码中执行没有球体出现,请修改代码
时间: 2024-02-14 11:03:12 浏览: 22
根据您提供的代码,我发现在 `create_sphere` 函数中,创建球体后没有将其添加到文档中。修改代码如下:
```python
import FreeCAD
import FreeCADGui
from PySide import QtGui, QtCore
Gui.activateWorkbench("PartWorkbench")
FreeCAD.newDocument()
class SphereDialog(QtGui.QDialog):
def __init__(self):
super().__init__()
self.setWindowTitle("Create Sphere")
self.create_widgets()
def create_widgets(self):
layout = QtGui.QVBoxLayout()
# Radius widget
radius_label = QtGui.QLabel("Radius:")
self.radius_spinbox = QtGui.QDoubleSpinBox()
self.radius_spinbox.setMinimum(0.1)
self.radius_spinbox.setMaximum(1000.0)
self.radius_spinbox.setValue(10.0)
layout.addWidget(radius_label)
layout.addWidget(self.radius_spinbox)
# Opacity widget
opacity_label = QtGui.QLabel("Opacity:")
self.opacity_slider = QtGui.QSlider(QtCore.Qt.Horizontal)
self.opacity_slider.setMinimum(0)
self.opacity_slider.setMaximum(100)
self.opacity_slider.setValue(50)
layout.addWidget(opacity_label)
layout.addWidget(self.opacity_slider)
# Create button
create_button = QtGui.QPushButton("Create")
create_button.clicked.connect(self.create_sphere)
layout.addWidget(create_button)
self.setLayout(layout)
def create_sphere(self):
# Get sphere parameters
radius = self.radius_spinbox.value()
opacity = float(self.opacity_slider.value() / 100)
# Create sphere
sphere = FreeCAD.ActiveDocument.addObject("Part::Sphere", "Sphere")
sphere.Radius = radius
sphere.ViewObject.Transparency = int(round(opacity * 100))
FreeCAD.ActiveDocument.recompute() # 添加这行代码,重新计算文档
# Show in 3D view
sphere.ViewObject.Visibility = True
FreeCADGui.SendMsgToActiveView("ViewFit")
FreeCADGui.Selection.clearSelection()
FreeCADGui.Selection.addSelection(sphere)
FreeCADGui.SendMsgToActiveView("ViewFit")
# Close dialog
self.close()
dialog = SphereDialog()
dialog.show()
```
在 `create_sphere` 函数中,我添加了 `FreeCAD.ActiveDocument.recompute()`,这会重新计算文档,使得添加的球体能够在 3D 视图中显示。
相关推荐
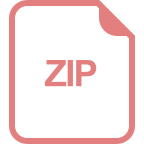
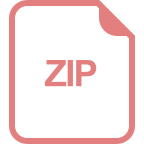
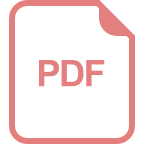














