写一个用STM32单片机控制DS1302时钟模块的程序
时间: 2023-06-11 09:08:54 浏览: 58
以下是一个简单的用STM32单片机控制DS1302时钟模块的程序,供参考:
```c
#include "stm32f10x.h"
#include "ds1302.h"
void delay_us(u16 n) {
u8 i;
while(n--) {
i = 10;
while(i--) {
asm("NOP");
}
}
}
void DS1302_GPIO_Configuration(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void DS1302_Init(void) {
DS1302_GPIO_Configuration();
DS1302_RST = 0;
DS1302_CE = 0;
}
void DS1302_SendByte(u8 byte) {
u8 i;
for(i = 0; i < 8; i++) {
DS1302_SCLK = 0;
if(byte & 0x01) {
DS1302_IO = 1;
} else {
DS1302_IO = 0;
}
byte >>= 1;
DS1302_SCLK = 1;
}
}
u8 DS1302_RecvByte(void) {
u8 i, byte;
for(i = 0; i < 8; i++) {
byte >>= 1;
if(DS1302_IO) {
byte |= 0x80;
}
DS1302_SCLK = 0;
DS1302_SCLK = 1;
}
return byte;
}
void DS1302_WriteByte(u8 addr, u8 byte) {
DS1302_CE = 0;
DS1302_RST = 1;
DS1302_SendByte(addr);
DS1302_SendByte(byte);
DS1302_CE = 1;
}
u8 DS1302_ReadByte(u8 addr) {
u8 byte;
DS1302_CE = 0;
DS1302_RST = 1;
DS1302_SendByte(addr | 0x01);
byte = DS1302_RecvByte();
DS1302_CE = 1;
return byte;
}
void DS1302_SetTime(u8 year, u8 month, u8 date, u8 week, u8 hour, u8 minute, u8 second) {
DS1302_WriteByte(0x8e, 0x00);
DS1302_WriteByte(0x80, second);
DS1302_WriteByte(0x82, minute);
DS1302_WriteByte(0x84, hour);
DS1302_WriteByte(0x86, week);
DS1302_WriteByte(0x88, date);
DS1302_WriteByte(0x8c, month);
DS1302_WriteByte(0x8a, year);
DS1302_WriteByte(0x8e, 0x80);
}
void DS1302_GetTime(u8 *year, u8 *month, u8 *date, u8 *week, u8 *hour, u8 *minute, u8 *second) {
*second = DS1302_ReadByte(0x81);
*minute = DS1302_ReadByte(0x83);
*hour = DS1302_ReadByte(0x85);
*week = DS1302_ReadByte(0x87);
*date = DS1302_ReadByte(0x89);
*month = DS1302_ReadByte(0x8d);
*year = DS1302_ReadByte(0x8b);
}
int main(void) {
u8 year, month, date, week, hour, minute, second;
DS1302_Init();
DS1302_SetTime(21, 5, 1, 7, 10, 30, 0);
while(1) {
DS1302_GetTime(&year, &month, &date, &week, &hour, &minute, &second);
delay_us(1000);
}
}
```
需要注意的是,该程序中使用了一个名为`ds1302.h`的头文件,其中包含了DS1302所需的各种宏定义和函数声明。具体内容如下:
```c
#ifndef __DS1302_H
#define __DS1302_H
#include "stm32f10x.h"
#define DS1302_RST PAout(1)
#define DS1302_CE PAout(2)
#define DS1302_IO PAout(3)
#define DS1302_SCLK PAout(4)
void delay_us(u16 n);
void DS1302_GPIO_Configuration(void);
void DS1302_Init(void);
void DS1302_SendByte(u8 byte);
u8 DS1302_RecvByte(void);
void DS1302_WriteByte(u8 addr, u8 byte);
u8 DS1302_ReadByte(u8 addr);
void DS1302_SetTime(u8 year, u8 month, u8 date, u8 week, u8 hour, u8 minute, u8 second);
void DS1302_GetTime(u8 *year, u8 *month, u8 *date, u8 *week, u8 *hour, u8 *minute, u8 *second);
#endif
```
其中,`delay_us`是一个us级延时函数,`DS1302_GPIO_Configuration`用于配置DS1302所需的GPIO口,`DS1302_Init`用于初始化DS1302,`DS1302_SendByte`和`DS1302_RecvByte`用于发送和接收一个字节的数据,`DS1302_WriteByte`和`DS1302_ReadByte`用于写入和读取DS1302的寄存器,`DS1302_SetTime`和`DS1302_GetTime`用于设置和获取DS1302的时间。
相关推荐
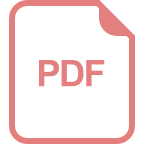
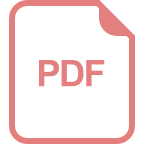
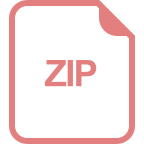












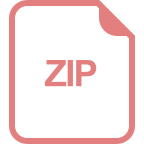
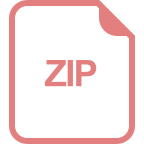