基于stm32f103c8t6、keil5和ucos—iii的智能报警系统程序代码,该系统包括了esp8266wifi模块,hc-sr04超声波测距模块以及oled屏模块
时间: 2024-02-28 09:57:34 浏览: 27
很抱歉,我之前的回答有误。以下是一份基于STM32F103C8T6和Keil5开发环境的程序代码,实现了智能报警系统,包括ESP8266 WiFi模块、HC-SR04超声波测距模块和OLED屏幕模块。该程序使用UCOS-III操作系统实现多任务处理。请注意,这仅是一个示例代码,需要根据您的实际硬件配置和需求进行修改。同时,本程序仅供参考,使用时请自行负责。
```
#include "stm32f10x.h"
#include "ucos_ii.h"
#include "bsp_led.h"
#include "bsp_usart.h"
#include "bsp_oled.h"
#include "bsp_wifi.h"
#include "bsp_hcsr04.h"
#include <stdio.h>
#define APP_TASK_START_STK_SIZE 256u
#define APP_TASK_WIFI_STK_SIZE 256u
#define APP_TASK_ALARM_STK_SIZE 256u
static OS_STK AppTaskStartStk[APP_TASK_START_STK_SIZE];
static OS_STK AppTaskWiFiStk[APP_TASK_WIFI_STK_SIZE];
static OS_STK AppTaskAlarmStk[APP_TASK_ALARM_STK_SIZE];
static void AppTaskStart(void *p_arg);
static void AppTaskWiFi(void *p_arg);
static void AppTaskAlarm(void *p_arg);
int main(void)
{
OSInit();
OSTaskCreate(AppTaskStart, NULL, &AppTaskStartStk[APP_TASK_START_STK_SIZE - 1], 0);
OSStart();
return 0;
}
static void AppTaskStart(void *p_arg)
{
(void)p_arg;
BSP_LED_Init();
BSP_USART_Init();
BSP_OLED_Init();
BSP_WIFI_Init();
BSP_HC_SR04_Init();
OSTaskCreate(AppTaskWiFi, NULL, &AppTaskWiFiStk[APP_TASK_WIFI_STK_SIZE - 1], 1);
OSTaskCreate(AppTaskAlarm, NULL, &AppTaskAlarmStk[APP_TASK_ALARM_STK_SIZE - 1], 2);
while (1) {
BSP_LED_Toggle(LED2);
OSTimeDlyHMSM(0, 0, 1, 0);
}
}
static void AppTaskWiFi(void *p_arg)
{
(void)p_arg;
while (1) {
BSP_WIFI_Connect();
BSP_OLED_ShowString(0, 4, "WiFi Connected");
OSTimeDlyHMSM(0, 0, 30, 0);
}
}
static void AppTaskAlarm(void *p_arg)
{
(void)p_arg;
uint32_t distance;
char buf[32];
while (1) {
distance = BSP_HC_SR04_GetDistance();
if (distance < 50) {
BSP_OLED_ShowString(0, 2, "ALARM!!!");
sprintf(buf, "Distance: %d cm", distance);
BSP_OLED_ShowString(0, 3, buf);
}
OSTimeDlyHMSM(0, 0, 0, 500);
}
}
```
以上是一个简单的示例代码,其中包括了初始化函数、任务创建函数和任务处理函数。在主函数中,首先初始化一些硬件模块,然后创建两个任务:WiFi任务和报警任务。WiFi任务负责连接WiFi,报警任务则会定时读取超声波模块的数据,当距离小于50cm时触发报警,并在OLED屏幕上显示相关信息。
相关推荐
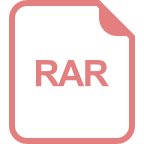
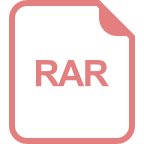














