float aspect_ratio = std::max(width, height) / min_value;
时间: 2023-06-18 17:02:02 浏览: 61
这行代码的作用是计算图像的宽高比(aspect ratio)。其中,`width` 和 `height` 是图像的宽度和高度,`min_value` 是一个常数,通常取两者之中的较小值。首先,通过 `std::max` 函数获取图像的最大边长。然后,将最大边长除以最小边长,得到的结果就是图像的宽高比。这个值可以用来判断图像的长宽比例是否符合要求,比如在计算机视觉领域中,有时需要对图像进行裁剪或缩放,这个值可以作为判断标准之一。
相关问题
请详细解释下这段代码Rect<float> FaceTracker::GetActiveBoundingRectangleOnActiveStream() const { std::vector<Rect<float>> faces = GetActiveFaceRectangles(); if (faces.empty()) { return Rect<float>(); } float min_x0 = 1.0f, min_y0 = 1.0f, max_x1 = 0.0f, max_y1 = 0.0f; for (const auto& f : faces) { min_x0 = std::min(f.left, min_x0); min_y0 = std::min(f.top, min_y0); max_x1 = std::max(f.right(), max_x1); max_y1 = std::max(f.bottom(), max_y1); } Rect<float> bounding_rect(min_x0, min_y0, max_x1 - min_x0, max_y1 - min_y0); VLOGF(2) << "Active bounding rect w.r.t active array: " << bounding_rect; // Transform the normalized rectangle in the active sensor array space to the // active stream space. const float active_array_aspect_ratio = static_cast<float>(options_.active_array_dimension.width) / static_cast<float>(options_.active_array_dimension.height); const float active_stream_aspect_ratio = static_cast<float>(options_.active_stream_dimension.width) / static_cast<float>(options_.active_stream_dimension.height); if (active_array_aspect_ratio < active_stream_aspect_ratio) { // The active stream is cropped into letterbox with smaller height than the // active sensor array. Adjust the y coordinates accordingly. const float height_ratio = active_array_aspect_ratio / active_stream_aspect_ratio; bounding_rect.height = std::min(bounding_rect.height / height_ratio, 1.0f); const float y_offset = (1.0f - height_ratio) / 2; bounding_rect.top = std::max(bounding_rect.top - y_offset, 0.0f) / height_ratio; } else { // The active stream is cropped into pillarbox with smaller width than the // active sensor array. Adjust the x coordinates accordingly. const float width_ratio = active_stream_aspect_ratio / active_array_aspect_ratio; bounding_rect.width = std::min(bounding_rect.width / width_ratio, 1.0f); const float x_offset = (1.0f - width_ratio) / 2; bounding_rect.left = std::max(bounding_rect.left - x_offset, 0.0f) / width_ratio; } VLOGF(2) << "Active bounding rect w.r.t active stream: " << bounding_rect; return bounding_rect; }
这段代码是一个类 FaceTracker 的成员函数 GetActiveBoundingRectangleOnActiveStream() 的实现。
该函数的作用是获取当前活动视频流中人脸的包围矩形,也就是所有人脸的最小矩形,返回一个 Rect<float> 类型的对象。
该函数的实现过程如下:
首先,调用 GetActiveFaceRectangles() 函数获取当前活动视频流中的所有人脸矩形,存储在 faces 变量中。
接着,判断 faces 是否为空,如果为空,则返回一个空的矩形(Rect<float>())。
如果 faces 不为空,则遍历 faces,计算所有人脸矩形的包围矩形,即最小的矩形,存储在 bounding_rect 变量中。
然后,根据 active_array_aspect_ratio 和 active_stream_aspect_ratio 计算出活动视频流的宽高比,判断活动视频流是被剪裁成了横向黑边或纵向黑边,进而计算出坐标的偏移量和缩放比例,将 bounding_rect 从活动传感器阵列空间转换为活动流空间,存储在 bounding_rect 变量中。
最后,返回 bounding_rect 变量。
函数中的 VLOGF(2) << ... 是一个日志输出语句,用于在输出日志时打印调试信息,2 表示日志输出级别为 verbose。
请详细解释下这段代码Rect<float> Framer::ComputeActiveCropRegion(int frame_number) { const float min_crop_size = 1.0f / options_.max_zoom_ratio; const float new_x_crop_size = std::clamp(region_of_interest_.width * options_.target_crop_to_roi_ratio, min_crop_size, 1.0f); const float new_y_crop_size = std::clamp(region_of_interest_.height * options_.target_crop_to_roi_ratio, min_crop_size, 1.0f); // We expand the raw crop region to match the desired output aspect ratio. const float target_aspect_ratio = static_cast<float>(options_.input_size.height) / static_cast<float>(options_.input_size.width) * static_cast<float>(options_.target_aspect_ratio_x) / static_cast<float>(options_.target_aspect_ratio_y); Rect<float> new_crop; if (new_x_crop_size <= new_y_crop_size * target_aspect_ratio) { new_crop.width = std::min(new_y_crop_size * target_aspect_ratio, 1.0f); new_crop.height = new_crop.width / target_aspect_ratio; } else { new_crop.height = std::min(new_x_crop_size / target_aspect_ratio, 1.0f); new_crop.width = new_crop.height * target_aspect_ratio; } const float roi_x_mid = region_of_interest_.left + (region_of_interest_.width / 2); const float roi_y_mid = region_of_interest_.top + (region_of_interest_.height / 2); new_crop.left = std::clamp(roi_x_mid - (new_crop.width / 2), 0.0f, 1.0f - new_crop.width); new_crop.top = std::clamp(roi_y_mid - (new_crop.height / 2), 0.0f, 1.0f - new_crop.height); const float normalized_crop_strength = std::powf(options_.crop_filter_strength, ElapsedTimeMs(timestamp_) / kUnitTimeSlice); active_crop_region_.left = IirFilter(active_crop_region_.left, new_crop.left, normalized_crop_strength); active_crop_region_.top = IirFilter(active_crop_region_.top, new_crop.top, normalized_crop_strength); active_crop_region_.width = IirFilter( active_crop_region_.width, new_crop.width, normalized_crop_strength); active_crop_region_.height = IirFilter( active_crop_region_.height, new_crop.height, normalized_crop_strength); timestamp_ = base::TimeTicks::Now(); if (VLOG_IS_ON(2)) { DVLOGFID(2, frame_number) << "region_of_interest=" << region_of_interest_; DVLOGFID(2, frame_number) << "new_crop_region=" << new_crop; DVLOGFID(2, frame_number) << "active_crop_region=" << active_crop_region_; } return active_crop_region_; }
这段代码是一个函数,函数名为ComputeActiveCropRegion,它接收一个整数frame_number作为参数,并返回一个Rect<float>类型的对象active_crop_region_。该函数的目的是计算当前帧的裁剪区域,并将其存储在active_crop_region_中,以便后续使用。
函数的实现过程如下:
首先,函数定义了一个常量min_crop_size,它表示最小的裁剪尺寸,即当裁剪区域的宽度或高度小于等于该值时,裁剪区域将不再缩小。然后,函数根据region_of_interest_的宽度和高度计算出新的裁剪区域的宽度和高度,这里使用了std::clamp函数将宽度和高度限制在[min_crop_size, 1.0f]的范围内。
接着,函数计算出目标宽高比target_aspect_ratio,该值是根据输入图像的尺寸和目标宽高比计算而来的。然后,函数根据新的裁剪区域的宽度和高度以及目标宽高比,计算出新的裁剪区域的左上角坐标和宽高。
最后,函数使用IIR滤波器将新的裁剪区域的左上角坐标和宽高与之前计算得到的active_crop_region_进行平滑处理,并将结果存储在active_crop_region_中。同时,函数还更新了timestamp_的值,用于计算IIR滤波器的时间间隔。
如果开启了VLOG(即日志调试),函数还会输出region_of_interest_、new_crop_region和active_crop_region_等信息。
总的来说,该函数的作用是计算当前帧的裁剪区域并将其平滑处理,以便后续使用。
相关推荐
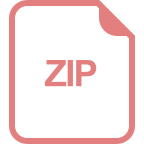
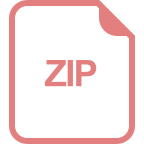












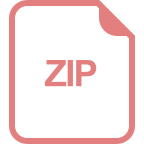