设计要求:必须使用面向对象的程序设计思想完成设计。 (1)某商家提供桌布定制服务,因为是定制的,所以桌布的价格是按照桌布的面积计算。不同型号的桌布单价是不同的,单价由商家提供。顾客定制时需提供桌布的宽与长,桌布的型号,依据桌布的面积计算桌布价格。但是桌布的型号输入错误,就认为是无效对象,显示“type 值 not exists”(请用异常来处理)。 (2)某顾客依据个人的需要定制了多块桌布,请列出该顾客的订单所有内容,并显示总件数与总价格。 输入:商家桌布型号与面积单价,整数n与n行桌布的型号、长度、宽度。 输出:若干块桌布的信息(型号、长度、宽度、桌布价格),总件数,总价格,其中,长度、宽度、价格保留一位小数。
时间: 2023-06-23 09:10:02 浏览: 88
以下是一个基于面向对象思想的 Python 实现:
```python
class Tablecloth:
def __init__(self, type, width, length, unit_price):
self.type = type
self.width = width
self.length = length
self.unit_price = unit_price
def area(self):
return self.width * self.length
def price(self):
return self.area() * self.unit_price
class Order:
def __init__(self, tablecloths):
self.tablecloths = tablecloths
def total_price(self):
return sum([t.price() for t in self.tablecloths])
def total_count(self):
return len(self.tablecloths)
if __name__ == '__main__':
prices = {}
n = int(input())
for i in range(n):
type, price = input().split()
prices[type] = float(price)
tablecloths = []
while True:
try:
type, width, length = input().split()
if type not in prices:
raise ValueError('type value not exists')
else:
tablecloth = Tablecloth(type, float(width), float(length), prices[type])
tablecloths.append(tablecloth)
except:
break
order = Order(tablecloths)
for t in tablecloths:
print('{} {} {} {:.1f}'.format(t.type, t.width, t.length, t.price()))
print('total count: {}, total price: {:.1f}'.format(order.total_count(), order.total_price()))
```
代码中定义了两个类,`Tablecloth` 表示桌布,包含了型号、宽度、长度和单价等属性,以及计算面积和价格的方法。`Order` 表示订单,包含了多个桌布对象,并提供了计算总价和总件数的方法。
在主程序中,首先读取商家提供的桌布型号和价格信息,并构建一个字典。然后循环读取顾客提供的桌布信息,每读入一块桌布,就创建一个 `Tablecloth` 对象,并将其添加到 `Order` 对象的桌布列表中。如果输入的型号不在商家提供的列表中,就抛出一个异常。
最后,输出每一块桌布的信息,以及总件数和总价格。
相关推荐
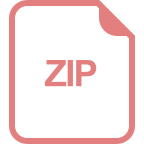
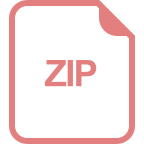
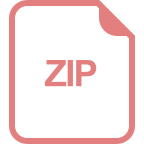








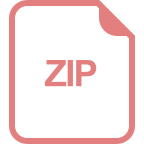
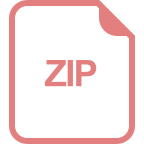
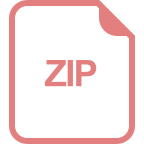
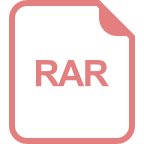
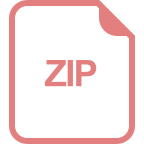
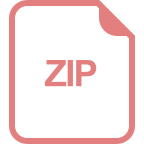