java实现时钟表盘教程方法
时间: 2023-07-28 22:10:39 浏览: 174
Java实现时钟表盘可以使用Java的Swing组件来绘制。下面是一个简单的示例:
```java
import javax.swing.*;
import java.awt.*;
public class ClockFace extends JPanel {
private int hours = 0;
private int minutes = 0;
private int seconds = 0;
private int radius = 0;
public ClockFace(int hours, int minutes, int seconds, int radius) {
this.hours = hours;
this.minutes = minutes;
this.seconds = seconds;
this.radius = radius;
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2 = (Graphics2D) g;
int width = getWidth();
int height = getHeight();
// 绘制表盘
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
g2.setColor(Color.WHITE);
g2.fillOval(0, 0, 2 * radius, 2 * radius);
// 绘制小时刻度
g2.setColor(Color.BLACK);
for (int i = 0; i < 12; i++) {
double angle = Math.PI / 6 * i;
int x1 = (int) (radius * Math.sin(angle) + radius);
int y1 = (int) (radius * Math.cos(angle) + radius);
int x2 = (int) ((radius - 15) * Math.sin(angle) + radius);
int y2 = (int) ((radius - 15) * Math.cos(angle) + radius);
g2.drawLine(x1, y1, x2, y2);
}
// 绘制分钟刻度
g2.setColor(Color.LIGHT_GRAY);
for (int i = 0; i < 60; i++) {
if (i % 5 != 0) {
double angle = Math.PI / 30 * i;
int x1 = (int) (radius * Math.sin(angle) + radius);
int y1 = (int) (radius * Math.cos(angle) + radius);
int x2 = (int) ((radius - 5) * Math.sin(angle) + radius);
int y2 = (int) ((radius - 5) * Math.cos(angle) + radius);
g2.drawLine(x1, y1, x2, y2);
}
}
// 绘制时针
g2.setColor(Color.BLACK);
double angle = Math.PI / 6 * ((hours % 12) + minutes / 60.0);
int x = (int) ((radius - 50) * Math.sin(angle) + radius);
int y = (int) ((radius - 50) * Math.cos(angle) + radius);
g2.setStroke(new BasicStroke(3.0f));
g2.drawLine(radius, radius, x, y);
// 绘制分针
g2.setColor(Color.BLACK);
angle = Math.PI / 30 * minutes;
x = (int) ((radius - 30) * Math.sin(angle) + radius);
y = (int) ((radius - 30) * Math.cos(angle) + radius);
g2.setStroke(new BasicStroke(2.0f));
g2.drawLine(radius, radius, x, y);
// 绘制秒针
g2.setColor(Color.RED);
angle = Math.PI / 30 * seconds;
x = (int) ((radius - 20) * Math.sin(angle) + radius);
y = (int) ((radius - 20) * Math.cos(angle) + radius);
g2.setStroke(new BasicStroke(1.0f));
g2.drawLine(radius, radius, x, y);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Clock");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 400);
frame.setLocationRelativeTo(null);
ClockFace clockFace = new ClockFace(12, 30, 0, 150);
frame.getContentPane().add(clockFace);
frame.setVisible(true);
}
}
```
ClockFace继承JPanel,并重写了paintComponent方法,该方法用于绘制时钟表盘。在构造函数中,传入了时、分、秒和表盘半径等参数。在paintComponent方法中,首先绘制了表盘圆形,然后绘制了小时刻度和分钟刻度。接着,根据时、分、秒计算出时针、分针和秒针的坐标,并绘制出来。在main方法中,创建一个JFrame窗口,并将ClockFace实例添加到窗口中。最后设置窗口的可见性即可。
阅读全文
相关推荐
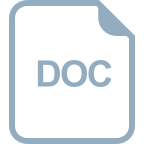
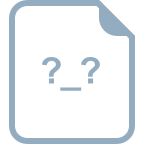
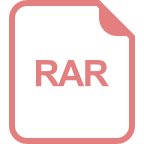
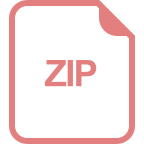
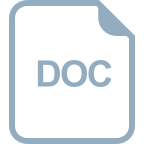
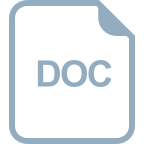
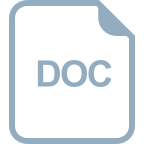
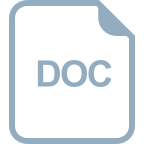
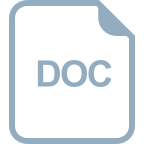
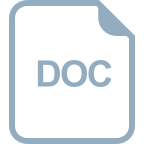
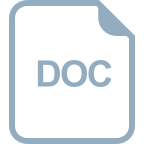
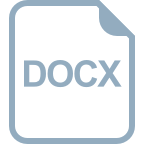