pyqt5设备图片中的特点形状
时间: 2023-11-22 13:34:34 浏览: 78
要识别设备图片中的特定形状,可以使用OpenCV和PyQt5的结合。具体的步骤如下:
1. 使用OpenCV读取图片
```python
import cv2
img = cv2.imread('image.png')
```
2. 对图像进行预处理和形状提取
可以使用OpenCV中的各种图像处理函数来对图像进行预处理和形状提取,比如边缘检测、轮廓检测等。
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
3. 在PyQt5中显示图像和形状
使用PyQt5中的QPixmap和QLabel来显示图像,并使用QPainter和QPen绘制形状。
```python
from PyQt5.QtGui import QPixmap, QImage, QPainter, QPen
from PyQt5.QtCore import Qt
from PyQt5.QtWidgets import QLabel
pixmap = QPixmap('image.png')
label = QLabel()
label.setPixmap(pixmap)
image = QImage(img.data, img.shape[1], img.shape[0], QImage.Format_RGB888).rgbSwapped()
painter = QPainter(image)
pen = QPen(Qt.red, 2, Qt.SolidLine)
painter.setPen(pen)
for contour in contours:
approx = cv2.approxPolyDP(contour, 0.01 * cv2.arcLength(contour, True), True)
if len(approx) == 4: # 识别矩形形状
x, y, w, h = cv2.boundingRect(approx)
painter.drawRect(x, y, w, h)
painter.end()
label.setPixmap(QPixmap.fromImage(image))
```
在上面的例子中,我们使用OpenCV中的`approxPolyDP()`函数来对轮廓进行多边形拟合,并判断拟合结果是否为矩形形状。如果是矩形,则使用QPainter类在图像上绘制矩形。最后,我们使用QLabel将绘制好的QPixmap显示在PyQt5窗口上。
以上是一个简单的流程,具体实现需要根据具体的需求来进行调整。
阅读全文
相关推荐
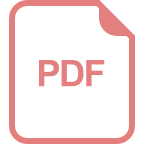
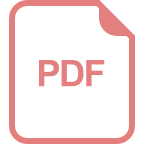
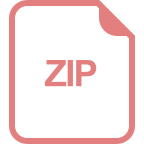
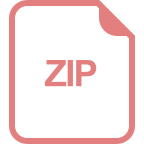
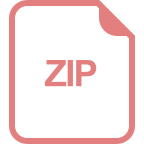
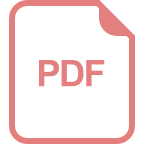
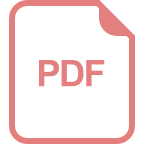
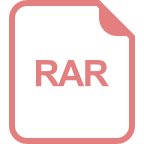
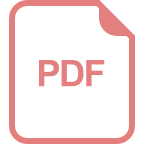
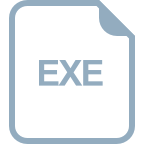
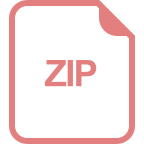
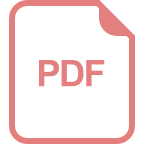