数码管 怎么用矩阵按键控制
时间: 2023-08-02 14:16:57 浏览: 92
使用矩阵按键控制数码管需要使用到微控制器或者单片机。以下是一种可能的实现步骤:
1. 连接数码管和矩阵按键到微控制器或单片机上。
2. 设置数码管的引脚为输出模式,设置矩阵按键的引脚为输入模式。
3. 初始化数码管和矩阵按键的引脚状态。
4. 在主循环中,检测矩阵按键的状态,如果有按键按下,则获取按键对应的数码,并显示在数码管上。
以下是一个示例代码,使用STM32F103C8T6单片机控制4位数码管和4x4矩阵按键:
```c
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
#define GPIO_PORT_NUM 4
#define GPIO_PIN_NUM 4
GPIO_TypeDef * const GPIO_PORT[GPIO_PORT_NUM] = {GPIOA, GPIOB, GPIOC, GPIOD};
const uint16_t GPIO_PIN[GPIO_PIN_NUM] = {GPIO_Pin_0, GPIO_Pin_1, GPIO_Pin_2, GPIO_Pin_3};
const uint8_t KEY_MAP[GPIO_PORT_NUM][GPIO_PIN_NUM] = {{1, 2, 3, 10}, {4, 5, 6, 11}, {7, 8, 9, 12}, {14, 0, 15, 13}};
void delay_us(uint32_t us)
{
uint32_t i;
for(i=0; i<us*8; ++i)
{
__ASM("NOP");
}
}
void GPIO_Init_All_Out(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB | RCC_APB2Periph_GPIOC | RCC_APB2Periph_GPIOD, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_All;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_Init(GPIOD, &GPIO_InitStructure);
}
void GPIO_Init_Key_In(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB | RCC_APB2Periph_GPIOC | RCC_APB2Periph_GPIOD, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_All;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_Init(GPIOD, &GPIO_InitStructure);
}
uint8_t Get_Key(void)
{
uint8_t i, j;
uint8_t key = 0;
for(i=0; i<GPIO_PORT_NUM; ++i)
{
GPIO_PORT[i]->BSRR = 0xFFFF << GPIO_PIN_NUM;
GPIO_PORT[i]->BRR = 1 << GPIO_PIN[i];
for(j=0; j<GPIO_PIN_NUM; ++j)
{
if((GPIO_PORT[i]->IDR & GPIO_PIN[j]) == 0)
{
key = KEY_MAP[i][j];
break;
}
}
GPIO_PORT[i]->BSRR = 0xFFFF << GPIO_PIN_NUM;
}
return key;
}
void Display_Digit(uint8_t digit)
{
uint8_t i;
for(i=0; i<4; ++i)
{
GPIO_PORT[i]->BSRR = 0xFFFF << GPIO_PIN_NUM;
GPIO_PORT[i]->BRR = (digit & (1 << i)) << GPIO_PIN[i];
}
delay_us(500);
}
int main(void)
{
uint8_t key = 0;
uint8_t digit = 0;
GPIO_Init_All_Out();
GPIO_Init_Key_In();
while(1)
{
key = Get_Key();
if(key > 0 && key <= 9)
{
digit = key;
}
else if(key == 10)
{
digit = 0;
}
Display_Digit(digit);
}
}
```
在上述代码中,使用GPIO口控制数码管和矩阵按键的状态,并轮询检测按键状态。当有按键按下时,获取对应的数字并显示在数码管上。需要注意的是,由于数码管是共阴极的,因此在显示数字时需要将对应的引脚设置为低电平,而矩阵按键是带上拉电阻的,因此设置为输入模式时需要启用上拉电阻。
相关推荐
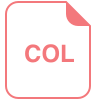
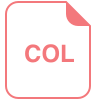
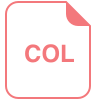
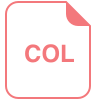
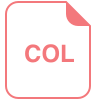









