请用C++实现一个链表,把rating.json文件数据读入到链表中,文件里的每条记录包含如下字段信息comment(int), from,comments,to,score,corp, time,有nlohmann库,样例记录为{ "comment": 0, "from": "北京-北京市-海淀区", "comments": [], "to": "河北省-承德市-隆化县", "score": "0", "corp": "兴铁物流", "time": "1398175318.988036" }
时间: 2023-08-22 19:09:18 浏览: 150
下面是一个简单的链表实现示例:
```c++
#include <iostream>
#include <fstream>
#include "nlohmann/json.hpp"
using namespace std;
using json = nlohmann::json;
// 定义链表节点
struct Node {
int comment;
string from;
vector<string> comments;
string to;
string score;
string corp;
string time;
Node* next;
};
// 读取json文件并返回json对象
json read_json_file(string filename) {
ifstream file(filename);
json j;
file >> j;
return j;
}
// 将json对象转换为链表
Node* json_to_list(json j) {
Node* head = nullptr;
Node* tail = nullptr;
for (auto it = j.begin(); it != j.end(); ++it) {
Node* node = new Node;
node->comment = it->at("comment");
node->from = it->at("from");
node->comments = it->at("comments");
node->to = it->at("to");
node->score = it->at("score");
node->corp = it->at("corp");
node->time = it->at("time");
node->next = nullptr;
if (head == nullptr) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
// 输出链表
void print_list(Node* head) {
while (head != nullptr) {
cout << head->comment << " " << head->from << " " << head->to << endl;
head = head->next;
}
}
int main() {
string filename = "rating.json";
json j = read_json_file(filename);
Node* head = json_to_list(j);
print_list(head);
return 0;
}
```
需要注意的是,这里使用了nlohmann库解析json文件,需要在编译时链接该库。例如,使用g++编译:
```
g++ -std=c++11 -o main main.cpp -ljsoncpp
```
阅读全文
相关推荐

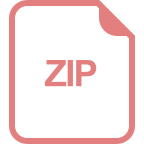
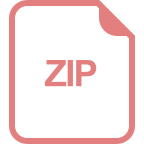
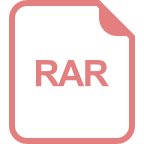
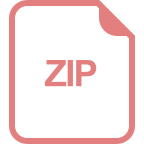
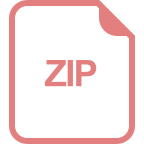
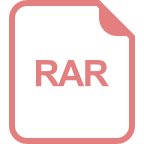
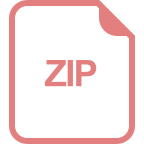
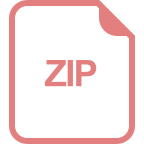
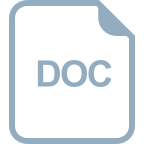
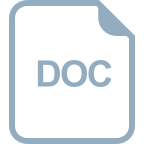
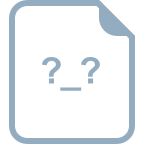
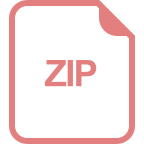
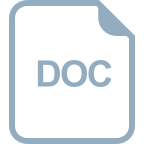