请使用多线程中的wait和notify实现字符串“***”和字符串“###”的严格交替输出,交替的之间休眠1秒: *** ### *** ### ,一定要严格交替,不能出现类似*** ### ### ***的交替.
时间: 2023-09-05 22:08:43 浏览: 46
使用多线程中的wait和notify,实现字符串“***”和字符串“___”交替输出可以通过以下方式:
1. 创建两个线程,分别打印字符串“***”和字符串“___”。
2. 在打印线程中,使用synchronized锁住公共变量,并在其中调用wait()方法。
3. 当一个线程打印完自己的字符串之后,调用notify()方法唤醒另一个线程。
4. 另一个线程被唤醒后,再次锁住公共变量并打印自己的字符串。重复以上步骤,实现交替输出。
示例代码如下:
```
public class PrintThread implements Runnable {
private String printStr;
private Object lock;
public PrintThread(String printStr, Object lock) {
this.printStr = printStr;
this.lock = lock;
}
@Override
public void run() {
synchronized (lock) {
for (int i = 0; i < 10; i++) {
try {
System.out.print(printStr);
lock.notify();
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
lock.notify();
}
}
}
public class PrintTest {
public static void main(String[] args) {
Object lock = new Object();
new Thread(new PrintThread("***", lock)).start(); // 打印 *** 的线程
new Thread(new PrintThread("___", lock)).start(); // 打印 ___ 的线程
}
}
```
相关推荐
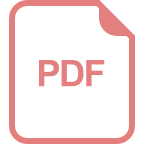
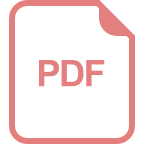
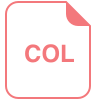













