Java实现短作业优先算法,PCB里需要有进程名,进程状态,运行时间和到达时间以及链表
时间: 2024-03-18 16:42:22 浏览: 12
以下是Java实现短作业优先算法的示例代码:
```java
// 定义进程类
class Process {
String name; // 进程名
int status; // 进程状态,0表示未到达,1表示已到达,2表示已完成
int runTime; // 运行时间
int arriveTime; // 到达时间
Process(String name, int runTime, int arriveTime) {
this.name = name;
this.status = 0;
this.runTime = runTime;
this.arriveTime = arriveTime;
}
}
// 定义链表节点类
class Node {
Process process; // 进程
Node next; // 下一个节点
Node(Process process) {
this.process = process;
this.next = null;
}
}
// 定义链表类
class LinkedList {
Node head; // 链表头节点
LinkedList() {
this.head = null;
}
// 插入节点
void insert(Process process) {
Node newNode = new Node(process);
if (head == null) {
head = newNode;
} else {
Node curr = head;
while (curr.next != null) {
curr = curr.next;
}
curr.next = newNode;
}
}
// 删除节点
void delete(Node node) {
if (node == head) {
head = head.next;
} else {
Node curr = head;
while (curr.next != node) {
curr = curr.next;
}
curr.next = node.next;
}
node.next = null;
}
// 获取链表长度
int length() {
int len = 0;
Node curr = head;
while (curr != null) {
len++;
curr = curr.next;
}
return len;
}
// 获取最短作业的节点
Node getShortestJob() {
Node curr = head;
Node shortestJob = head;
while (curr != null) {
if (curr.process.status == 1 && curr.process.runTime < shortestJob.process.runTime) {
shortestJob = curr;
}
curr = curr.next;
}
return shortestJob;
}
}
public class SJF {
public static void main(String[] args) {
// 初始化进程列表
LinkedList processList = new LinkedList();
processList.insert(new Process("P1", 3, 0));
processList.insert(new Process("P2", 2, 1));
processList.insert(new Process("P3", 1, 2));
processList.insert(new Process("P4", 4, 3));
processList.insert(new Process("P5", 2, 4));
// 运行进程
int time = 0;
while (processList.length() > 0) {
Node shortestJob = processList.getShortestJob();
if (shortestJob.process.status == 1) {
System.out.println("Time " + time + ": " + shortestJob.process.name + " is running");
shortestJob.process.runTime--;
time++;
if (shortestJob.process.runTime == 0) {
System.out.println("Time " + time + ": " + shortestJob.process.name + " is finished");
shortestJob.process.status = 2;
processList.delete(shortestJob);
}
} else {
time++;
}
}
}
}
```
在上面的代码中,我们定义了进程类 `Process`,包含进程名、进程状态、运行时间和到达时间等属性;定义了链表节点类 `Node`,包含进程和下一个节点的引用;定义了链表类 `LinkedList`,包含头节点、插入节点、删除节点、获取链表长度和获取最短作业的节点等方法。最后,在 `main` 方法中初始化进程列表,然后按照最短作业优先算法运行进程,直到所有进程都完成为止。
相关推荐
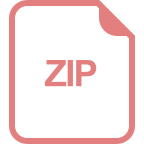
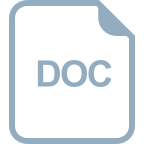
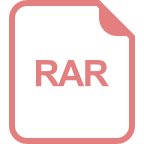














