一、设计一个小型公司的信息管理系统,内容如下。 某小型公司主要有4类人:经理、兼职技术人员、销售经理、兼职销售员,这些人 具有以下属性: 经理:姓名、编号、级别、固定工资、当月薪水、计算月薪、显示信息。 兼职技术人员:姓名、编号、级别、工作小时、每小时工资额、当月薪水、计算月 薪、显示信息。 兼职销售员:姓名、编号、级别、销售额、销售额提成、当月薪水、计算月薪、显 示信息。 销售经理:姓名、编号、级别、固定工资、销售额、销售额提成、当月薪水、计算 月薪、显示信息。 要求: 1、 人员编号要求基数为1000,每输入一个人员信息编号顺序加1; 2、 要有主函数,在主函数里定义不同类的对象,实现人员信息的输出。 3、月薪计算办法: 经理固定月薪8000元;兼职技术人员按100元/小时领取月薪;兼职推销员按当月销 售额的4%提成;销售经理固定月薪5000,销售提成为所管辖部门当月销售总额的千 分之五。用c++编写
时间: 2023-07-10 14:43:01 浏览: 105
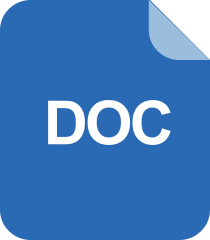
用C++编程——小型公司工资管理系统.doc
好的,我可以帮你设计一个小型公司的信息管理系统,以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 声明类
class Manager;
class PartTimeTech;
class PartTimeSales;
class SalesManager;
// 定义人员类
class Person {
protected:
string name;
int id;
int level;
float salary;
public:
static int base_id;
Person(string n, int l, float s) {
name = n;
level = l;
salary = s;
id = base_id++;
}
virtual float calcSalary() = 0;
virtual void displayInfo() = 0;
};
// 定义经理类
class Manager: public Person {
private:
float month_salary;
public:
Manager(string n, int l, float s): Person(n, l, s) {
month_salary = calcSalary();
}
float calcSalary() {
return 8000;
}
void displayInfo() {
cout << "姓名:" << name << endl;
cout << "编号:" << id << endl;
cout << "级别:" << level << endl;
cout << "固定工资:" << salary << endl;
cout << "当月薪水:" << month_salary << endl;
}
};
// 定义兼职技术人员类
class PartTimeTech: public Person {
private:
float work_hour;
float hour_salary;
float month_salary;
public:
PartTimeTech(string n, int l, float s, float wh, float hs): Person(n, l, s) {
work_hour = wh;
hour_salary = hs;
month_salary = calcSalary();
}
float calcSalary() {
return work_hour * hour_salary;
}
void displayInfo() {
cout << "姓名:" << name << endl;
cout << "编号:" << id << endl;
cout << "级别:" << level << endl;
cout << "工作小时:" << work_hour << endl;
cout << "每小时工资额:" << hour_salary << endl;
cout << "当月薪水:" << month_salary << endl;
}
};
// 定义兼职销售员类
class PartTimeSales: public Person {
private:
float sales;
float commission;
float month_salary;
public:
PartTimeSales(string n, int l, float s, float sl, float c): Person(n, l, s) {
sales = sl;
commission = c;
month_salary = calcSalary();
}
float calcSalary() {
return sales * commission;
}
void displayInfo() {
cout << "姓名:" << name << endl;
cout << "编号:" << id << endl;
cout << "级别:" << level << endl;
cout << "销售额:" << sales << endl;
cout << "销售额提成:" << commission << endl;
cout << "当月薪水:" << month_salary << endl;
}
};
// 定义销售经理类
class SalesManager: public Person {
private:
float sales;
float commission;
float month_salary;
public:
SalesManager(string n, int l, float s, float sl, float c): Person(n, l, s) {
sales = sl;
commission = c;
month_salary = calcSalary();
}
float calcSalary() {
return salary + sales * commission * 0.005;
}
void displayInfo() {
cout << "姓名:" << name << endl;
cout << "编号:" << id << endl;
cout << "级别:" << level << endl;
cout << "固定工资:" << salary << endl;
cout << "销售额:" << sales << endl;
cout << "销售额提成:" << commission << endl;
cout << "当月薪水:" << month_salary << endl;
}
};
// 初始化编号
int Person::base_id = 1000;
int main() {
// 创建不同类的对象
Manager m("张三", 1, 10000);
PartTimeTech ptt("李四", 2, 0, 80, 100);
PartTimeSales pts("王五", 3, 0, 100000, 0.04);
SalesManager sm("赵六", 4, 5000, 200000, 0.05);
// 输出人员信息
m.displayInfo();
cout << endl;
ptt.displayInfo();
cout << endl;
pts.displayInfo();
cout << endl;
sm.displayInfo();
return 0;
}
```
注意:以上代码只是一个简单的示例,实际开发中还需要根据需求进行完善和优化。
阅读全文
相关推荐
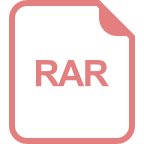
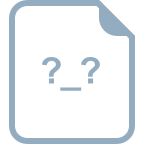



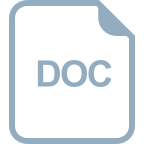
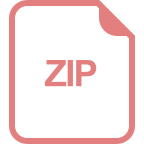
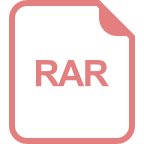
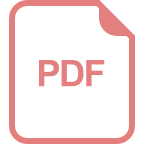
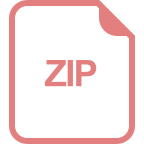
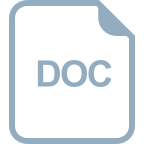
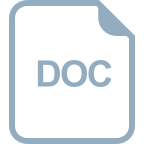
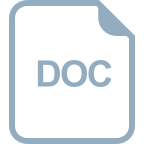
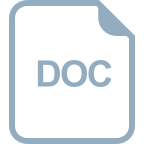
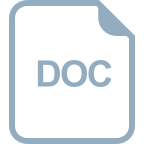
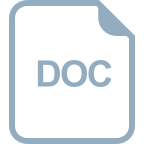
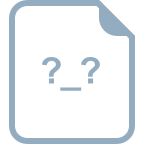