学校图书馆新进一批图书,请用C语言编写软件以存储这些图书的信息。图书信息主要指:图书名称,出版社,数量,单价。软件应具备以下主要功能:(1) 数据输入。即,能够输入图书信息。(2) 数据保存。即,能够将输入的信息以文件的形式保存到计算机中。(3) 数据查询。即,能够按照“图书名称”查询图书信息,也能够按照“单价”查询图书信息。(4) 数据修改。即,当输入数据出现误操作时,事后可以更改。软件至少应具有“数据输入”、“保存”、“查询”、“修改”、“退出”5项菜单。
时间: 2024-03-30 11:35:39 浏览: 16
好的,以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct book {
char name[50];
char publisher[50];
int quantity;
float price;
};
void save_books(struct book *books, int num_books);
void load_books(struct book *books, int *num_books);
void add_book(struct book *books, int *num_books);
void search_book(struct book *books, int num_books);
void modify_book(struct book *books, int num_books);
int main() {
struct book books[MAX_BOOKS];
int num_books = 0;
int choice;
load_books(books, &num_books);
do {
printf("\nMenu:\n");
printf("1. Add book\n");
printf("2. Save books\n");
printf("3. Search book\n");
printf("4. Modify book\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book(books, &num_books);
break;
case 2:
save_books(books, num_books);
break;
case 3:
search_book(books, num_books);
break;
case 4:
modify_book(books, num_books);
break;
case 5:
break;
default:
printf("Invalid choice\n");
}
} while (choice != 5);
return 0;
}
void save_books(struct book *books, int num_books) {
FILE *fp;
int i;
fp = fopen("books.dat", "wb");
if (fp == NULL) {
printf("Error creating file\n");
return;
}
fwrite(&num_books, sizeof(int), 1, fp);
fwrite(books, sizeof(struct book), num_books, fp);
fclose(fp);
printf("%d books saved to file\n", num_books);
}
void load_books(struct book *books, int *num_books) {
FILE *fp;
int i;
fp = fopen("books.dat", "rb");
if (fp == NULL) {
printf("File not found\n");
return;
}
fread(num_books, sizeof(int), 1, fp);
fread(books, sizeof(struct book), *num_books, fp);
fclose(fp);
printf("%d books loaded from file\n", *num_books);
}
void add_book(struct book *books, int *num_books) {
struct book new_book;
printf("Enter book name: ");
scanf("%s", new_book.name);
printf("Enter publisher: ");
scanf("%s", new_book.publisher);
printf("Enter quantity: ");
scanf("%d", &new_book.quantity);
printf("Enter price: ");
scanf("%f", &new_book.price);
books[*num_books] = new_book;
(*num_books)++;
printf("Book added\n");
}
void search_book(struct book *books, int num_books) {
int choice;
char name[50];
float price;
int i;
printf("Search by:\n");
printf("1. Book name\n");
printf("2. Price\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter book name: ");
scanf("%s", name);
for (i = 0; i < num_books; i++) {
if (strcmp(books[i].name, name) == 0) {
printf("Book found:\n");
printf("Name: %s\n", books[i].name);
printf("Publisher: %s\n", books[i].publisher);
printf("Quantity: %d\n", books[i].quantity);
printf("Price: %.2f\n", books[i].price);
return;
}
}
printf("Book not found\n");
break;
case 2:
printf("Enter price: ");
scanf("%f", &price);
for (i = 0; i < num_books; i++) {
if (books[i].price == price) {
printf("Book found:\n");
printf("Name: %s\n", books[i].name);
printf("Publisher: %s\n", books[i].publisher);
printf("Quantity: %d\n", books[i].quantity);
printf("Price: %.2f\n", books[i].price);
return;
}
}
printf("Book not found\n");
break;
default:
printf("Invalid choice\n");
}
}
void modify_book(struct book *books, int num_books) {
char name[50];
int i;
printf("Enter book name to modify: ");
scanf("%s", name);
for (i = 0; i < num_books; i++) {
if (strcmp(books[i].name, name) == 0) {
printf("Enter new book name: ");
scanf("%s", books[i].name);
printf("Enter new publisher: ");
scanf("%s", books[i].publisher);
printf("Enter new quantity: ");
scanf("%d", &books[i].quantity);
printf("Enter new price: ");
scanf("%f", &books[i].price);
printf("Book modified\n");
return;
}
}
printf("Book not found\n");
}
```
这个程序使用结构体来存储每本书的信息,使用文件来保存和读取图书信息。用户可以选择添加新书、保存图书信息、查询图书信息、修改图书信息或退出程序。在查询和修改图书信息时,可以按照书名或单价进行搜索。
相关推荐
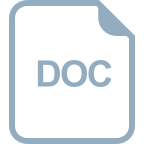
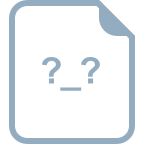
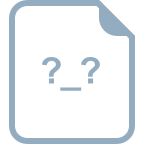














