Python gradio.DataFrame()怎么接受pandas.DataFrame
时间: 2023-11-03 07:00:46 浏览: 525
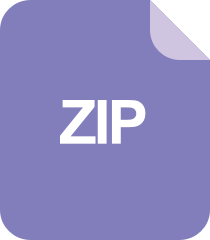
gradio-master.zip

Gradio的DataFrame()函数可以接受pandas.DataFrame,只需要将pandas.DataFrame对象传递给DataFrame()函数即可。下面是一个简单的示例代码:
```
import gradio as gr
import pandas as pd
# 创建pandas.DataFrame对象
data = {'Name': ['Bob', 'Jane', 'Alice'],
'Age': [25, 30, 35],
'Country': ['USA', 'Canada', 'UK']}
df = pd.DataFrame(data)
# 使用Gradio的DataFrame()函数展示pandas.DataFrame
interface = gr.Interface(fn=lambda df: df, inputs="pandas.DataFrame", outputs="dataframe")
interface.test_launch()
```
在上面的代码中,我们首先创建了一个pandas.DataFrame对象df,然后使用Gradio的DataFrame()函数展示了该数据框。注意,我们在Gradio的Interface()函数中将输入类型设为"pandas.DataFrame",输出类型设为"dataframe"。
阅读全文
相关推荐
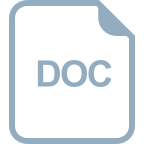
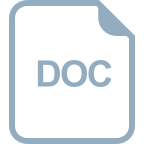

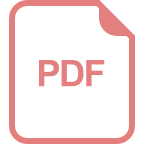












