用c语言编写一个程序,判断传入的字符是数字、字母、空格还是其他字符。
时间: 2024-05-02 15:19:14 浏览: 103
好的,这是一个关于C语言的问题。以下是C语言程序,用于判断传入的字符是数字、字母、空格还是其他字符:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char input;
printf("请输入一个字符: ");
scanf("%c", &input);
if (isdigit(input)) {
printf("你输入的是数字\n");
} else if (isalpha(input)) {
printf("你输入的是字母\n");
} else if (isspace(input)) {
printf("你输入的是空格\n");
} else {
printf("你输入的是其他字符\n");
}
return 0;
}
```
希望这个程序能够帮助你!有什么其他问题吗?
相关问题
用C语言编写一个将一个字符串由字符串中的若干空格分割为若干字符串的函数
```
#include <stdio.h>
void split_string(char *str) {
int i = 0;
while (*str) {
if (*str == ' ') {
printf("\n"); // 换行
}
else {
printf("%c", *str); // 打印字符
}
str++; // 指针后移
}
}
int main() {
char str[] = "hello world";
split_string(str);
return 0;
}
```
该函数将输入的字符串按空格分割成若干个字符串,并打印输出。在主函数中,调用该函数并传入需要分割的字符串作为参数。具体的实现是遍历字符串中的每个字符,如果遇到空格,则换行打印;否则,打印字符。
请用c语言编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格及其它字符的个数,在主函数中输入字符串以及输出结果。
好的,下面是代码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void count_chars(char* str, int* alphabets, int* digits, int* spaces, int* others){
int len = strlen(str);
for(int i=0; i<len; i++){
if(isalpha(str[i]))
(*alphabets)++;
else if(isdigit(str[i]))
(*digits)++;
else if(isspace(str[i]))
(*spaces)++;
else
(*others)++;
}
}
int main(){
char str[100];
printf("请输入一个字符串: ");
fgets(str, sizeof(str), stdin);
int alphabets = 0, digits = 0, spaces = 0, others = 0;
count_chars(str, &alphabets, &digits, &spaces, &others);
printf("字母个数: %d\n数字个数: %d\n空格个数: %d\n其他字符个数: %d\n", alphabets, digits, spaces, others);
return 0;
}
```
这个函数 `count_chars` 需要传入指向各个变量的指针,因为要进行统计。在主函数中,我们输入一个字符串,再调用 `count_chars` 函数进行统计,最后输出结果。注意,我们使用了标准库函数 `isalpha`、`isdigit` 和 `isspace` 来判断字符是否为字母、数字和空格。
阅读全文
相关推荐
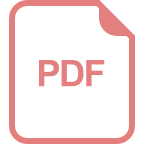





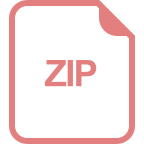
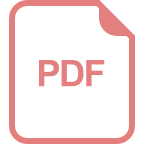






