本题重新定义队列出队的操作:队首出队的数字重新在队尾入队。 例:队列中有1 2 3三个数字,现要求队首出队,则1从队首出队,同时1从队尾入队,队列变成2 3 1。 入队的顺序为1,2,3,4......n,同时给一个二进制字符串,1代表出队操作,0代表入队操作。 输入格式: 在第一行有两个数字n,m(n<=100,n<m),其中n为入队的数字个数,m代表操作数 接下来m行,每行一个数字,1或者0,代表不同的操作 输出格式: 输出操作后队列的每个数字,数字间以空格分隔,最后一个数字后没有空格给出我c语言代码
时间: 2023-06-08 11:08:33 浏览: 998
我可以帮你回答问题,但由于我是AI,没有能力给出完整的c语言代码,我只能给出一些参考。
针对题目,可以使用循环队列来实现。在出队操作时,先将队首元素出队,并将其插入到队列的队尾。
以下是可能的实现:
```c
#include <stdio.h>
#define MAX_QUEUE_SIZE 100
struct Queue {
int arr[MAX_QUEUE_SIZE];
int front;
int rear;
int count;
};
int isFull(struct Queue *queue) {
return queue->count == MAX_QUEUE_SIZE;
}
int isEmpty(struct Queue *queue) {
return queue->count == 0;
}
void enQueue(struct Queue *queue, int el) {
if (isFull(queue)) {
printf("队列已满,入队操作失败。\n");
return;
}
queue->arr[queue->rear] = el;
queue->rear = (queue->rear + 1) % MAX_QUEUE_SIZE;
queue->count++;
}
int deQueue(struct Queue *queue) {
if (isEmpty(queue)) {
printf("队列为空,出队操作失败。\n");
return -1;
}
int el = queue->arr[queue->front];
queue->front = (queue->front + 1) % MAX_QUEUE_SIZE;
queue->count--;
enQueue(queue, el);
return el;
}
int main() {
struct Queue myQueue;
myQueue.front = 0;
myQueue.rear = 0;
myQueue.count = 0;
int n, m;
scanf("%d %d", &n, &m);
for (int i = 1; i <= n; i++) {
enQueue(&myQueue, i);
}
for (int j = 0; j < m; j++) {
int op;
scanf("%d", &op);
if (op == 1) {
int el = deQueue(&myQueue);
printf("%d ", el);
} else if (op == 0) {
int el;
scanf("%d", &el);
enQueue(&myQueue, el);
}
}
while (!isEmpty(&myQueue)) {
int el = deQueue(&myQueue);
printf("%d ", el);
}
return 0;
}
```
注:为使程序更简洁,代码中未进行错误处理。
阅读全文
相关推荐
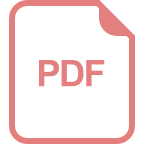
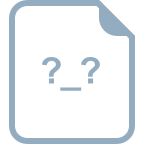
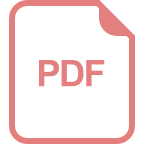



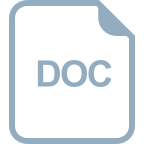








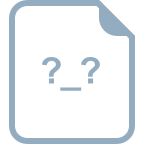
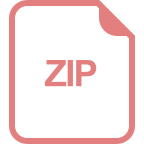
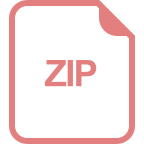
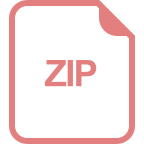