用c语言写一个字符串格式化代码,要求所有的换行转成空格,不允许出现连续空格
时间: 2023-04-04 13:03:48 浏览: 56
你好,以下是我的回答:
可以使用C语言中的字符串处理函数来实现字符串格式化。具体实现方法如下:
1. 定义一个字符串变量,用于存储原始字符串和格式化后的字符串。
2. 使用fgets函数从标准输入中读取原始字符串。
3. 使用strtok函数将原始字符串按照换行符分割成多个子字符串。
4. 使用strcat函数将每个子字符串连接起来,并在子字符串之间添加一个空格。
5. 使用strtok函数将格式化后的字符串按照空格分割成多个子字符串。
6. 使用循环遍历每个子字符串,如果当前子字符串不为空,则将其添加到最终的格式化字符串中,并在子字符串之间添加一个空格。
7. 最后输出格式化后的字符串。
以下是示例代码:
#include <stdio.h>
#include <string.h>
int main()
{
char str[1000] = {0};
char *token = NULL;
char *delim = "\n";
char *space = " ";
char *format_str = NULL;
printf("请输入原始字符串:\n");
fgets(str, sizeof(str), stdin);
// 将换行符替换成空格
token = strtok(str, delim);
while (token != NULL)
{
strcat(format_str, token);
strcat(format_str, space);
token = strtok(NULL, delim);
}
// 将连续空格替换成一个空格
token = strtok(format_str, space);
while (token != NULL)
{
if (strlen(token) > 0)
{
strcat(str, token);
strcat(str, space);
}
token = strtok(NULL, space);
}
printf("格式化后的字符串为:%s\n", str);
return 0;
}
相关推荐
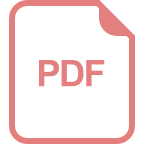
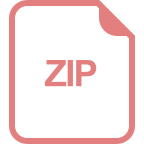
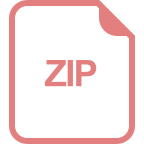














