Android studio计算器代码
时间: 2023-09-23 08:04:15 浏览: 55
以下是一个简单的 Android Studio 计算器代码,支持加减乘除和清零操作:
1. 在 XML 文件中创建 UI 布局:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/resultTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="textEnd"
android:textSize="24sp"
android:padding="16dp"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/buttonClear"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="C"/>
<Button
android:id="@+id/buttonDivide"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="/"/>
<Button
android:id="@+id/buttonMultiply"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="*"/>
<Button
android:id="@+id/buttonDelete"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="DEL"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/buttonSeven"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="7"/>
<Button
android:id="@+id/buttonEight"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="8"/>
<Button
android:id="@+id/buttonNine"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="9"/>
<Button
android:id="@+id/buttonMinus"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="-"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/buttonFour"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="4"/>
<Button
android:id="@+id/buttonFive"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="5"/>
<Button
android:id="@+id/buttonSix"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="6"/>
<Button
android:id="@+id/buttonPlus"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="+"/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/buttonOne"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="1"/>
<Button
android:id="@+id/buttonTwo"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="2"/>
<Button
android:id="@+id/buttonThree"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="3"/>
<Button
android:id="@+id/buttonEqual"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="="/>
</LinearLayout>
<Button
android:id="@+id/buttonZero"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="0"/>
</LinearLayout>
```
2. 在 Java 文件中添加计算逻辑:
```java
public class MainActivity extends AppCompatActivity {
private TextView resultTextView;
private String operand1 = "";
private String operand2 = "";
private String operator = "";
private String result = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
resultTextView = findViewById(R.id.resultTextView);
Button buttonClear = findViewById(R.id.buttonClear);
Button buttonDivide = findViewById(R.id.buttonDivide);
Button buttonMultiply = findViewById(R.id.buttonMultiply);
Button buttonDelete = findViewById(R.id.buttonDelete);
Button buttonSeven = findViewById(R.id.buttonSeven);
Button buttonEight = findViewById(R.id.buttonEight);
Button buttonNine = findViewById(R.id.buttonNine);
Button buttonMinus = findViewById(R.id.buttonMinus);
Button buttonFour = findViewById(R.id.buttonFour);
Button buttonFive = findViewById(R.id.buttonFive);
Button buttonSix = findViewById(R.id.buttonSix);
Button buttonPlus = findViewById(R.id.buttonPlus);
Button buttonOne = findViewById(R.id.buttonOne);
Button buttonTwo = findViewById(R.id.buttonTwo);
Button buttonThree = findViewById(R.id.buttonThree);
Button buttonEqual = findViewById(R.id.buttonEqual);
Button buttonZero = findViewById(R.id.buttonZero);
buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operand1 = "";
operand2 = "";
operator = "";
result = "";
resultTextView.setText(result);
}
});
buttonDivide.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operator = "/";
if (!operand1.equals("")) {
resultTextView.setText(operand1 + operator);
}
}
});
buttonMultiply.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operator = "*";
if (!operand1.equals("")) {
resultTextView.setText(operand1 + operator);
}
}
});
buttonDelete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 = operand1.substring(0, operand1.length() - 1);
resultTextView.setText(operand1);
} else {
operand2 = operand2.substring(0, operand2.length() - 1);
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonSeven.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "7";
resultTextView.setText(operand1);
} else {
operand2 += "7";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonEight.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "8";
resultTextView.setText(operand1);
} else {
operand2 += "8";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonNine.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "9";
resultTextView.setText(operand1);
} else {
operand2 += "9";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonMinus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operator = "-";
if (!operand1.equals("")) {
resultTextView.setText(operand1 + operator);
}
}
});
buttonFour.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "4";
resultTextView.setText(operand1);
} else {
operand2 += "4";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonFive.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "5";
resultTextView.setText(operand1);
} else {
operand2 += "5";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonSix.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "6";
resultTextView.setText(operand1);
} else {
operand2 += "6";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonPlus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
operator = "+";
if (!operand1.equals("")) {
resultTextView.setText(operand1 + operator);
}
}
});
buttonOne.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "1";
resultTextView.setText(operand1);
} else {
operand2 += "1";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonTwo.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "2";
resultTextView.setText(operand1);
} else {
operand2 += "2";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonThree.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "3";
resultTextView.setText(operand1);
} else {
operand2 += "3";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
buttonEqual.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!operand1.equals("") && !operand2.equals("") && !operator.equals("")) {
double d1 = Double.parseDouble(operand1);
double d2 = Double.parseDouble(operand2);
if (operator.equals("/")) {
result = String.valueOf(d1 / d2);
} else if (operator.equals("*")) {
result = String.valueOf(d1 * d2);
} else if (operator.equals("-")) {
result = String.valueOf(d1 - d2);
} else if (operator.equals("+")) {
result = String.valueOf(d1 + d2);
}
resultTextView.setText(result);
operand1 = result;
operand2 = "";
operator = "";
}
}
});
buttonZero.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (operator.equals("")) {
operand1 += "0";
resultTextView.setText(operand1);
} else {
operand2 += "0";
resultTextView.setText(operand1 + operator + operand2);
}
}
});
}
}
```
以上就是 Android Studio 计算器的简单实现。注意,此处没有进行错误处理,例如用户输入非法字符或除数为零等情况。
相关推荐
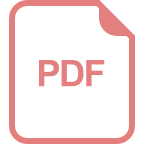














