用mysql和python写一个图书管理系统
时间: 2024-04-29 19:22:00 浏览: 133
好的,这是一个比较大的项目,我会简单介绍一下如何实现这个系统,希望能帮到你。
首先,需要安装mysql和python的相关模块。推荐使用MySQLdb模块,它可以让你在python中操作mysql数据库。
接着,需要设计数据库,包括表结构和字段。这个系统需要考虑到书籍的基本信息,如书名、作者、出版社、价格等等,同时也需要考虑到借阅者信息,如姓名、手机号、借书时间等等。可以设计两个表,一个是书籍信息表,另一个是借阅者信息表。
然后,需要编写python代码,实现对数据库的增删改查操作。可以定义一个Book类和Reader类,分别对应书籍信息和借阅者信息。在类中定义相关属性和方法,并通过MySQLdb模块实现对数据库的操作。
最后,可以编写一个简单的用户界面,让用户可以方便地进行图书管理。可以使用Tkinter模块实现界面设计,通过按钮和输入框等控件实现用户界面。
当然,这只是一个简单的实现思路,具体的实现还需要考虑到更多细节问题,如数据校验、异常处理等等。希望这些信息能对你有所帮助。
相关问题
帮我用mysql+python写一个图书管理数据库系统
好的,这是一个比较复杂的项目,需要涉及到MySQL数据库的设计和Python编程。以下是一个简单的实现过程示例。
1. 数据库设计
首先,我们需要设计一个数据库,以存储图书信息和借阅记录。以下是一个简单的数据库设计:
- 图书表(Book)
- id: 图书编号
- title: 标题
- author: 作者
- publisher: 出版社
- pub_date: 出版日期
- price: 价格
- total: 总数量
- current: 当前数量
- 借阅记录表(Borrow)
- id: 记录编号
- book_id: 图书编号
- user_id: 用户编号
- borrow_date: 借阅日期
- return_date: 归还日期
2. Python程序实现
接下来,我们可以使用Python编写一个简单的程序,以与数据库交互。
首先,我们需要安装MySQL Python驱动程序。你可以使用以下命令:
```
pip install mysql-connector-python
```
然后,我们需要编写Python程序,以实现以下功能:
- 添加图书信息
- 修改图书信息
- 删除图书信息
- 查询图书信息
- 借阅图书
- 归还图书
- 查询借阅记录
以下是一个简单的Python程序示例:
```python
import mysql.connector
# 连接数据库
db = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# 创建游标对象
cursor = db.cursor()
# 添加图书信息
def add_book(title, author, publisher, pub_date, price, total, current):
sql = "INSERT INTO Book (title, author, publisher, pub_date, price, total, current) VALUES (%s, %s, %s, %s, %s, %s, %s)"
val = (title, author, publisher, pub_date, price, total, current)
cursor.execute(sql, val)
db.commit()
# 修改图书信息
def update_book(id, title, author, publisher, pub_date, price, total, current):
sql = "UPDATE Book SET title = %s, author = %s, publisher = %s, pub_date = %s, price = %s, total = %s, current = %s WHERE id = %s"
val = (title, author, publisher, pub_date, price, total, current, id)
cursor.execute(sql, val)
db.commit()
# 删除图书信息
def delete_book(id):
sql = "DELETE FROM Book WHERE id = %s"
val = (id,)
cursor.execute(sql, val)
db.commit()
# 查询图书信息
def search_book(title=None, author=None, publisher=None, pub_date=None):
sql = "SELECT * FROM Book WHERE 1=1"
if title:
sql += " AND title = %s"
val = (title,)
if author:
sql += " AND author = %s"
val = (author,)
if publisher:
sql += " AND publisher = %s"
val = (publisher,)
if pub_date:
sql += " AND pub_date = %s"
val = (pub_date,)
cursor.execute(sql, val)
result = cursor.fetchall()
for row in result:
print(row)
# 借阅图书
def borrow_book(book_id, user_id, borrow_date):
sql = "INSERT INTO Borrow (book_id, user_id, borrow_date) VALUES (%s, %s, %s)"
val = (book_id, user_id, borrow_date)
cursor.execute(sql, val)
db.commit()
sql = "UPDATE Book SET current = current - 1 WHERE id = %s"
val = (book_id,)
cursor.execute(sql, val)
db.commit()
# 归还图书
def return_book(id, return_date):
sql = "UPDATE Borrow SET return_date = %s WHERE id = %s"
val = (return_date, id)
cursor.execute(sql, val)
db.commit()
sql = "SELECT book_id FROM Borrow WHERE id = %s"
val = (id,)
cursor.execute(sql, val)
result = cursor.fetchone()
book_id = result[0]
sql = "UPDATE Book SET current = current + 1 WHERE id = %s"
val = (book_id,)
cursor.execute(sql, val)
db.commit()
# 查询借阅记录
def search_borrow(user_id=None, book_id=None):
sql = "SELECT * FROM Borrow WHERE 1=1"
if user_id:
sql += " AND user_id = %s"
val = (user_id,)
if book_id:
sql += " AND book_id = %s"
val = (book_id,)
cursor.execute(sql, val)
result = cursor.fetchall()
for row in result:
print(row)
# 测试代码
add_book("Python编程从入门到实践", "Eric Matthes", "人民邮电出版社", "2016-11-01", 98.0, 10, 10)
add_book("流浪地球", "刘慈欣", "重庆出版社", "2010-10-01", 42.0, 5, 5)
search_book(author="刘慈欣")
borrow_book(1, 1, "2021-01-01")
search_borrow()
return_book(1, "2021-01-10")
search_borrow()
```
以上代码是一个简单的图书管理系统示例,您可以根据自己的需求进行修改和扩展。
使用python和mysql写一个图书管理系统
好的,下面是一个简单的图书管理系统的Python代码,它连接到MySQL数据库并允许用户添加、删除和更新图书信息:
```python
import mysql.connector
# 连接到MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="library"
)
# 创建游标对象
mycursor = mydb.cursor()
# 定义函数以添加新书
def add_book(title, author, year, isbn):
sql = "INSERT INTO books (title, author, year, isbn) VALUES (%s, %s, %s, %s)"
values = (title, author, year, isbn)
mycursor.execute(sql, values)
mydb.commit()
print("书籍已成功添加!")
# 定义函数以查找书籍
def search_book(title="", author="", year="", isbn=""):
sql = "SELECT * FROM books WHERE title=%s OR author=%s OR year=%s OR isbn=%s"
values = (title, author, year, isbn)
mycursor.execute(sql, values)
results = mycursor.fetchall()
return results
# 定义函数以更新书籍信息
def update_book(id, title, author, year, isbn):
sql = "UPDATE books SET title=%s, author=%s, year=%s, isbn=%s WHERE id=%s"
values = (title, author, year, isbn, id)
mycursor.execute(sql, values)
mydb.commit()
print("书籍信息已成功更新!")
# 定义函数以删除书籍
def delete_book(id):
sql = "DELETE FROM books WHERE id=%s"
values = (id,)
mycursor.execute(sql, values)
mydb.commit()
print("书籍已成功删除!")
# 添加书籍
add_book("Python入门", "张三", "2020", "1234567890")
# 查找书籍
results = search_book(author="张三")
for result in results:
print(result)
# 更新书籍信息
update_book(1, "Python基础", "李四", "2021", "0987654321")
# 删除书籍
delete_book(1)
```
在上面的代码中,我们首先连接到名为“library”的MySQL数据库。然后,我们定义了四个函数,分别用于添加、查找、更新和删除图书信息。每个函数都使用MySQL连接来执行相应的SQL查询。最后,我们测试了每个函数并输出了结果。请注意,在上面的代码中,我们使用了MySQL连接器模块,该模块需要通过pip安装。
阅读全文
相关推荐
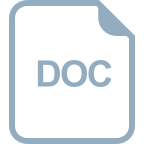
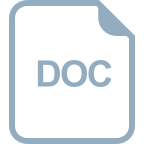
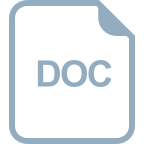













