java中能将字符串解析运算符的
时间: 2023-12-28 18:18:34 浏览: 122
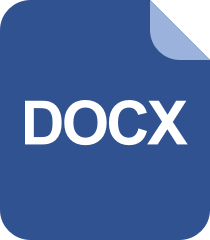
Java 73道基础面试题.docx
在 Java 中可以使用以下方法将字符串解析为运算符:
1. 使用 switch 语句对字符串进行匹配,然后执行相应的操作。例如:
```
String operator = "+";
int operand1 = 2;
int operand2 = 3;
switch (operator) {
case "+":
System.out.println(operand1 + operand2);
break;
case "-":
System.out.println(operand1 - operand2);
break;
case "*":
System.out.println(operand1 * operand2);
break;
case "/":
System.out.println(operand1 / operand2);
break;
default:
System.out.println("Invalid operator");
}
```
2. 使用 Java 中的反射机制,根据字符串动态获取对应的运算符类或方法,并执行相应的操作。例如:
```
String operator = "+";
int operand1 = 2;
int operand2 = 3;
try {
Class<?> operatorClass = Class.forName("java.lang." + operator);
Method operatorMethod = operatorClass.getMethod("apply", int.class, int.class);
Object result = operatorMethod.invoke(null, operand1, operand2);
System.out.println(result);
} catch (Exception e) {
System.out.println("Invalid operator");
}
```
在这个例子中,我们假设存在一个名为 `java.lang. +` 的类,该类中包含一个名为 `apply` 的方法,接受两个 int 类型的参数并返回它们的和。使用反射机制,我们可以根据字符串 `"+"` 动态获取该类及其方法,并执行相应的操作。当然,这只是一个示例,实际中并不存在名为 `java.lang. +` 的类。
阅读全文
相关推荐
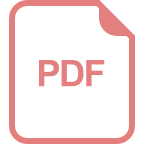
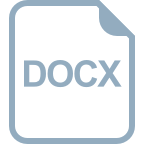
















