用Opencv编写一个图像梯度Sobel的算法
时间: 2024-05-05 21:21:49 浏览: 107
我们可以使用OpenCV中提供的Sobel函数实现图像梯度Sobel算法。以下是使用C++编写的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像
Mat image = imread("test.jpg");
// 转换为灰度图像
Mat grayImage;
cvtColor(image, grayImage, COLOR_BGR2GRAY);
// 计算x方向梯度
Mat grad_x;
Sobel(grayImage, grad_x, CV_16S, 1, 0);
// 计算y方向梯度
Mat grad_y;
Sobel(grayImage, grad_y, CV_16S, 0, 1);
// 转换为8位无符号整型
Mat abs_grad_x;
convertScaleAbs(grad_x, abs_grad_x);
Mat abs_grad_y;
convertScaleAbs(grad_y, abs_grad_y);
// 合并梯度图像
Mat gradImage;
addWeighted(abs_grad_x, 0.5, abs_grad_y, 0.5, 0, gradImage);
// 显示梯度图像
namedWindow("Sobel Image", WINDOW_NORMAL);
imshow("Sobel Image", gradImage);
waitKey(0);
return 0;
}
```
在代码中,我们首先读取一张图像并将其转换为灰度图像。然后使用Sobel函数分别计算x方向和y方向的梯度。由于Sobel函数计算出来的是有符号的16位整型数,因此需要使用convertScaleAbs函数将其转换为8位无符号整型。最后将x方向和y方向的梯度图像按照一定比例融合在一起,得到最终的梯度图像。
阅读全文
相关推荐
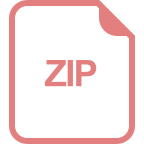
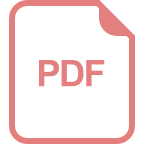

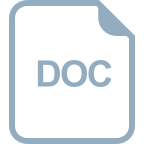
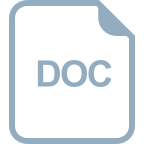

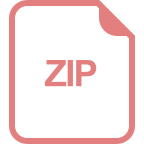
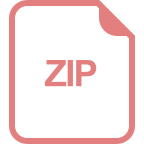
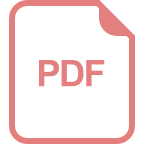
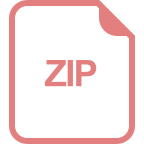
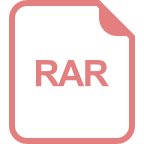
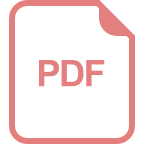
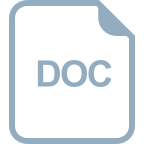
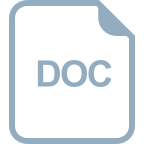
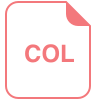

