java实现多个类对象读取和存储文件
时间: 2024-05-06 10:18:20 浏览: 10
可以通过以下步骤实现多个类对象读取和存储文件:
1. 定义一个包含文件读写方法的工具类,例如FileUtils。该类应该包含读取和写入文件的静态方法,例如readFile和writeFile。
2. 在每个类中创建一个实例变量来保存文件路径,例如String filePath。
3. 在每个类的构造函数中初始化filePath变量并加载文件内容到对象中。可以使用FileUtils类中的readFile方法来实现。
4. 在每个类中定义一个save方法,用于将对象的属性写入到文件中。可以使用FileUtils类中的writeFile方法来实现。
5. 在应用程序中创建多个类的实例,并调用它们的save方法以将它们的属性保存到文件中。
以下是一个示例代码:
```java
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class FileUtils {
public static String readFile(String filePath) throws IOException {
// 读取文件内容
}
public static void writeFile(String filePath, String content) throws IOException {
// 写入文件内容
}
}
public class Person {
private String name;
private int age;
private String filePath;
public Person(String name, int age, String filePath) throws IOException {
this.name = name;
this.age = age;
this.filePath = filePath;
loadFromFile();
}
public void save() throws IOException {
String content = name + "," + age;
FileUtils.writeFile(filePath, content);
}
private void loadFromFile() throws IOException {
String content = FileUtils.readFile(filePath);
String[] parts = content.split(",");
this.name = parts[0];
this.age = Integer.parseInt(parts[1]);
}
}
public class Main {
public static void main(String[] args) throws IOException {
List<Person> persons = new ArrayList<>();
persons.add(new Person("Alice", 20, "alice.txt"));
persons.add(new Person("Bob", 30, "bob.txt"));
for (Person person : persons) {
person.save();
}
}
}
```
在上面的示例中,FileUtils类包含了读取和写入文件的静态方法。Person类保存了一个文件路径,并在构造函数中使用loadFromFile方法将文件内容加载到对象中。save方法将对象的属性写入到文件中。在Main类中,我们创建了两个Person对象并将它们保存到文件中。
相关推荐
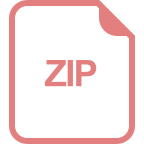
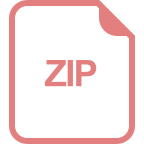
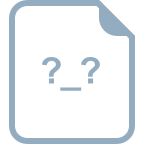














