qt 怎么实现tcp连接不成功自动重连?断开自动重连?
时间: 2024-01-27 11:03:30 浏览: 22
Qt 可以使用 `QTcpSocket` 类来实现 TCP 连接,通过监听 `errorOccurred` 信号和 `disconnected` 信号,我们可以实现连接失败自动重连和断开连接自动重连的功能。
下面是一个示例代码:
```cpp
#include <QTcpSocket>
#include <QTimer>
class TcpClient : public QObject {
Q_OBJECT
public:
TcpClient(const QString& host, int port, QObject* parent = nullptr)
: QObject(parent)
, m_host(host)
, m_port(port)
{
connect(&m_socket, &QTcpSocket::connected, this, &TcpClient::onConnected);
connect(&m_socket, &QTcpSocket::disconnected, this, &TcpClient::onDisconnected);
connect(&m_socket, &QTcpSocket::errorOccurred, this, &TcpClient::onErrorOccurred);
connect(&m_reconnectTimer, &QTimer::timeout, this, &TcpClient::reconnect);
connect(&m_socket, &QTcpSocket::readyRead, this, &TcpClient::onReadyRead);
connectToServer();
}
signals:
void messageReceived(const QString& message);
private slots:
void connectToServer()
{
m_socket.connectToHost(m_host, m_port);
}
void onConnected()
{
m_reconnectTimer.stop();
qDebug() << "Connected to" << m_host << ":" << m_port;
}
void onDisconnected()
{
qDebug() << "Disconnected from" << m_host << ":" << m_port;
m_reconnectTimer.start(5000); // 5秒后尝试重新连接
}
void onErrorOccurred(QAbstractSocket::SocketError error)
{
qWarning() << "TCP socket error:" << error;
m_reconnectTimer.start(5000); // 5秒后尝试重新连接
}
void reconnect()
{
qDebug() << "Reconnecting to" << m_host << ":" << m_port;
connectToServer();
}
void onReadyRead()
{
QString message = QString::fromUtf8(m_socket.readAll());
emit messageReceived(message);
}
private:
QTcpSocket m_socket;
QString m_host;
int m_port;
QTimer m_reconnectTimer;
};
```
在上面的代码中,我们创建了一个 `TcpClient` 类来处理 TCP 连接。在构造函数中,我们连接了 `connected` 信号、`disconnected` 信号、`errorOccurred` 信号和 `readyRead` 信号,分别对应连接成功、连接断开、连接错误和收到数据的情况。在 `connectToServer()` 函数中,我们调用 `m_socket.connectToHost()` 来连接服务器。在 `onDisconnected()` 和 `onErrorOccurred()` 函数中,我们启动了一个 `QTimer` 来在一定时间后尝试重新连接服务器。
注意,这个示例只是一个简单的示例,实际的应用可能需要更加复杂的处理逻辑,例如限制重连次数、增加重连时间间隔等。
相关推荐
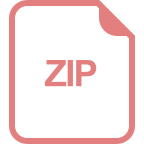
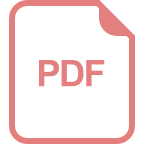














